请用js写一个页面,页面为一个表单,第一行表单可以输入文本区域,第二个表单可以上传radio文件,第三个表单可以上传和显示图片,第四个表单可以上传显示和下载附件。
时间: 2024-06-10 17:09:02 浏览: 126
好的,这是一个很简单的任务,可以使用HTML和JavaScript来完成。
首先,我们需要在HTML文件中创建一个表单,如下所示:
```html
<form>
<label for="text-input">文本输入:</label>
<textarea id="text-input"></textarea>
<label for="file-input">文件上传:</label>
<input type="file" id="file-input">
<label for="image-input">图片上传:</label>
<input type="file" id="image-input">
<img id="image-preview">
<label for="attachment-input">附件上传:</label>
<input type="file" id="attachment-input">
<a href="#" id="attachment-link" download>下载附件</a>
<button type="submit">提交</button>
</form>
```
这个表单包括四个表单元素:一个文本输入框、一个文件上传框、一个图片上传框和一个附件上传框。此外,我们还添加了一个提交按钮。
然后,我们需要编写一些JavaScript代码来实现这些表单元素的功能。下面是完整的代码:
```javascript
// 获取表单元素
const textInput = document.getElementById('text-input');
const fileInput = document.getElementById('file-input');
const imageInput = document.getElementById('image-input');
const imagePreview = document.getElementById('image-preview');
const attachmentInput = document.getElementById('attachment-input');
const attachmentLink = document.getElementById('attachment-link');
// 监听文件上传框的change事件
fileInput.addEventListener('change', () => {
const file = fileInput.files[0];
console.log(file);
});
// 监听图片上传框的change事件
imageInput.addEventListener('change', () => {
const file = imageInput.files[0];
const reader = new FileReader();
reader.addEventListener('load', () => {
imagePreview.src = reader.result;
});
reader.readAsDataURL(file);
});
// 监听附件上传框的change事件
attachmentInput.addEventListener('change', () => {
const file = attachmentInput.files[0];
attachmentLink.href = URL.createObjectURL(file);
attachmentLink.download = file.name;
});
// 监听表单的submit事件
document.querySelector('form').addEventListener('submit', (event) => {
event.preventDefault();
// 获取文本输入框的值
const textValue = textInput.value;
console.log(textValue);
// 获取文件上传框的值
const file = fileInput.files[0];
console.log(file);
// 获取图片上传框的值
const image = imagePreview.src;
console.log(image);
// 获取附件上传框的值
const attachment = attachmentInput.files[0];
console.log(attachment);
// 提交表单
// ...
});
```
在这段代码中,我们首先获取了表单元素,然后分别为文件上传框、图片上传框和附件上传框添加了change事件监听器,当用户选择文件后,我们可以获取文件对象并进行相应的处理。对于图片上传框,我们使用了FileReader API将图片转换为base64编码的字符串,并在页面上显示预览图。对于附件上传框,我们使用了URL.createObjectURL()方法将文件对象转换为URL,并设置下载链接的href属性和download属性。最后,在表单提交时,我们获取了各个表单元素的值,并可以进行相应的处理。
希望这个例子可以帮助你了解如何使用JavaScript创建表单。如果你还有其它问题,请随时问我!
阅读全文
相关推荐




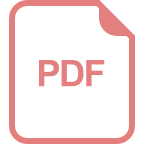
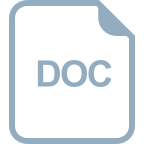









