如何在Python中编写一个简单的银行ATM模拟程序?
时间: 2024-11-24 09:40:08 浏览: 14
在Python中编写一个简单的银行ATM模拟程序,可以按照以下步骤进行:
1. **初始化**:创建一个类`BankATM`,包含账户相关的属性(如账户名、余额、密码等)和方法(如登录、存钱、取钱、查询余额)。
```python
class BankATM:
def __init__(self, account_name, balance, password):
self.account_name = account_name
self.balance = balance
self.password = password
# 添加其他方法...
```
2. **登录验证**:在`login`方法中检查输入的密码是否匹配。
```python
def login(self, entered_password):
if entered_password == self.password:
return True
else:
return False
```
3. **基本操作**:为每个核心功能(如`deposit`, `withdraw`和`check_balance`)编写函数,包括处理用户输入、校验、更新余额等。
```python
def deposit(self, amount):
if self.login() and amount > 0:
self.balance += amount
print(f"已成功存入 {amount} 元,当前余额为 {self.balance} 元")
else:
print("操作失败")
# 类似地添加 withdraw 和 check_balance 方法...
```
4. **主循环**:创建一个用户界面,让用户体验操作过程。
```python
def main():
atm = BankATM("John Doe", 1000, "password123")
while True:
action = input("请输入您的操作(deposit, withdraw, check_balance, quit):")
if action.lower() == "quit":
break
elif action.lower() in ["deposit", "withdraw", "check_balance"]:
# 调用相应的 ATM 功能
# ...
else:
print("无效操作")
if __name__ == "__main__":
main()
```
这个例子仅做演示,实际应用中会涉及更复杂的安全措施和异常处理机制。
阅读全文
相关推荐
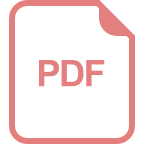
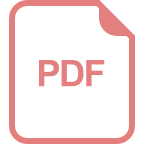
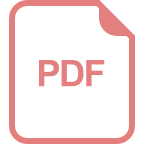
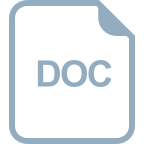
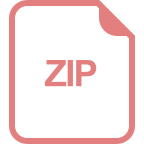





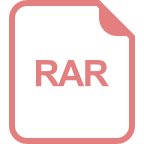
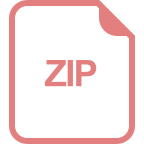
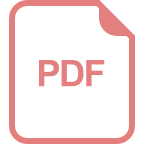
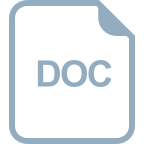




