#include <stdio.h> #include <string.h> #include <stdlib.h>是什么
时间: 2023-11-18 08:03:34 浏览: 446
这是C语言中的头文件,用于包含一些常用的函数和宏定义。具体来说:
- stdio.h:包含了输入输出函数,例如printf和scanf。
- string.h:包含了字符串处理函数,例如strcpy和strcat。
- stdlib.h:包含了一些常用的函数,例如malloc和free,以及一些常用的宏定义,例如EXIT_SUCCESS和EXIT_FAILURE。
这些头文件可以让我们在编写C程序时更加方便地使用这些函数和宏定义,而不需要自己重新实现。如果你想使用这些函数或宏定义,只需要在程序中包含相应的头文件即可。
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#include<stdio.h>#include<stdlib.h>#include<string.h>
在C语言中,`#include<stdio.h>`、`#include<stdlib.h>`和`#include<string.h>`分别包含了输入/输出、内存管理以及字符串处理相关的库。这里给你演示如何使用这些头文件中的函数:
1. **输入/输出(stdio.h)**:
```c
#include<stdio.h>
int main() {
printf("Hello, World!\n"); // 使用printf输出字符串
scanf("%s", "Enter your name: "); // 使用scanf从用户那里读取输入
return 0;
}
```
2. **内存管理(stdlib.h)**:
```c
#include<stdlib.h>
int *createArray(int size) {
int *array = malloc(size * sizeof(int)); // 分配动态内存
return array;
}
void freeMemory(int *array, int size) {
free(array); // 释放已分配的内存
}
```
3. **字符串处理(string.h)**:
```c
#include<string.h>
char* copyString(const char* src) {
char* dest = (char*)malloc(strlen(src) + 1); // 动态分配内存复制字符串
strcpy(dest, src);
return dest;
}
```
注意,C语言的标准库函数可能需要链接到适当的库,如`-lstdc++` 或 `-lm`,具体取决于编译器设置。
阅读全文
相关推荐
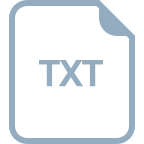
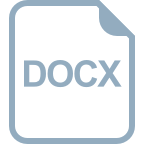
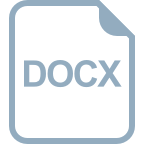













