mybatis的resultMap的id是必要的吗
时间: 2024-05-30 16:10:04 浏览: 2
是的,resultMap的id是必要的。在MyBatis中,每个resultMap必须有一个唯一的id来标识它。这个id在其他地方被引用,例如在mapper文件中使用<select>语句时,需要使用resultMap的id来指定结果映射。如果没有为resultMap指定id,MyBatis无法识别它,并会抛出异常。因此,为每个resultMap定义一个唯一的id是必要的。
相关问题
mybatis的mapper.xml resultMap怎么定义数组
在 MyBatis 的 `resultMap` 中,如果要定义数组类型的属性,可以使用 `collection` 元素来表示。例如,假设我们有一个 `User` 对象,其中有一个 `String[] hobbies` 属性,我们可以通过以下方式在 `resultMap` 中定义:
```xml
<resultMap id="userResultMap" type="User">
<id property="id" column="id"/>
<result property="name" column="name"/>
<collection property="hobbies" ofType="String">
<result column="hobby"/>
</collection>
</resultMap>
```
在上面的示例中,`collection` 元素指定了 `property` 属性为 `hobbies`,表示要将查询结果中 `hobby` 列的值映射到 `User` 对象的 `hobbies` 属性中。`ofType` 属性指定了 `hobbies` 属性的元素类型为 `String`。注意,`collection` 元素必须放在 `resultMap` 中的 `id` 和 `result` 元素之后,否则会导致解析错误。
mybatis返回一个数组的时候resultmap怎么填
如果MyBatis返回一个数组,可以使用以下方式填写resultMap:
1. 使用resultType属性,指定返回值类型为数组类型,例如:
```
<select id="selectUsers" resultType="com.example.User[]">
select * from user
</select>
```
2. 使用resultMap属性,使用resultMap引用一个已经定义好的resultMap,例如:
```
<resultMap id="userMap" type="com.example.User">
<id property="id" column="user_id" />
<result property="name" column="user_name" />
<result property="age" column="user_age" />
</resultMap>
<select id="selectUsers" resultMap="userMap">
select * from user
</select>
```
需要注意的是,在resultMap中定义的属性必须和返回的数组元素类型的属性名一致,否则会导致映射失败。
相关推荐
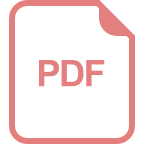
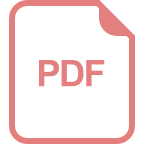
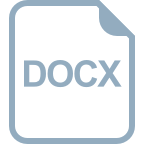
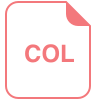
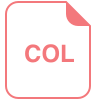
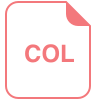
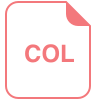
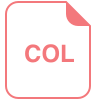







