Please provide python code for generating a data file with a random distribution of polymer chains for lammps
时间: 2023-02-08 11:12:12 浏览: 231
这里是一个示例代码,可以帮助您生成随机分布的聚合物链的数据文件:
```
import random
# 设定聚合物的数量和链长
num_polymers = 10
polymer_length = 100
# 创建一个空的列表,用于存储聚合物的坐标
polymers = []
# 随机生成聚合物的坐标
for i in range(num_polymers):
polymer = []
for j in range(polymer_length):
x = random.uniform(-10, 10)
y = random.uniform(-10, 10)
z = random.uniform(-10, 10)
polymer.append((x, y, z))
polymers.append(polymer)
# 打开文件并写入数据
with open('polymers.data', 'w') as f:
# 写入聚合物的数量和链长
f.write(f'{num_polymers} {polymer_length}\n')
# 写入每条聚合物的坐标
for polymer in polymers:
for x, y, z in polymer:
f.write(f'{x} {y} {z}\n')
```
这段代码会生成一个名为 `polymers.data` 的数据文件,其中包含了随机生成的聚合物的坐标。您可以根据需要调整聚合物的数量和链长的值。
相关问题
Collecting BeautifulSoup Using cached BeautifulSoup-3.2.2.tar.gz (32 kB) Preparing metadata (setup.py) ... error error: subprocess-exited-with-error × python setup.py egg_info did not run successfully. │ exit code: 1 ╰─> [7 lines of output] Traceback (most recent call last): File "<string>", line 2, in <module> File "<pip-setuptools-caller>", line 34, in <module> File "C:\Users\Lenovo\AppData\Local\Temp\pip-install-wwckn9qw\beautifulsoup_cd386e3e7ae14b8988153f9c919e4f80\setup.py", line 3 "You're trying to run a very old release of Beautiful Soup under Python 3. This will not work."<>"Please use Beautiful Soup 4, available through the pip package 'beautifulsoup4'." ^^ SyntaxError: invalid syntax [end of output] note: This error originates from a subprocess, and is likely not a problem with pip. error: metadata-generation-failed × Encountered error while generating package metadata. ╰─> See above for output. note: This is an issue with the package mentioned above, not pip. hint: See above for details.
这个错误提示是在安装 BeautifulSoup 时出现的。根据错误提示,你正在尝试在 Python 3 下运行一个非常旧的版本的 Beautiful Soup,这是无法工作的。建议你使用 Beautiful Soup 4,可以通过 pip 包 'beautifulsoup4' 安装。你可以使用以下命令安装 Beautiful Soup 4:
```
pip install beautifulsoup4
```
安装完成后,你可以在 Python 中导入 Beautiful Soup 进行使用。
• You are required to justify your choice of number of clusters by doing a proper elbow analysis and including the code for generating the elbow plot as part of the submitted R script. • Use the k-means method since it makes the “total within sum of squares’ ’ metric readily available as part of the model output. • Reuse the same datasets and script/solution from assignment 2 in order to produce the dataframe that contains 2018 data on healthcare spending and life expectancy (this should be the starting point for this assignment).
Sure, I can help you with that! Here's how you can perform an elbow analysis to determine the optimal number of clusters for your k-means model:
1. Load the necessary libraries and data:
```
library(tidyverse)
library(cluster)
df <- read.csv("healthcare_data.csv", header = TRUE)
```
2. Clean and prepare the data by selecting only the relevant columns and removing any missing values:
```
df_clean <- df %>% select(Life.Expectancy, Healthcare.Spending) %>% drop_na()
```
3. Scale the data to ensure that both variables have equal influence on the clustering:
```
df_scaled <- scale(df_clean)
```
4. Run the k-means algorithm for a range of cluster values (e.g. 1 to 10) and calculate the total within-cluster sum of squares (WSS) for each:
```
wss <- sapply(1:10, function(k){
kmeans(df_scaled, k, nstart = 10)$tot.withinss
})
```
5. Plot the WSS values against the number of clusters and identify the "elbow" point where adding more clusters does not significantly reduce the WSS:
```
plot(1:10, wss, type = "b", pch = 19, frame = FALSE,
xlab = "Number of clusters K", ylab = "Total within-clusters sum of squares")
```
6. Based on the plot, select the optimal number of clusters for your k-means model. The "elbow" point is usually where the curve starts to flatten out, indicating diminishing returns from adding more clusters.
Here's the complete R code for performing an elbow analysis on the healthcare spending and life expectancy data:
```
library(tidyverse)
library(cluster)
# Load data
df <- read.csv("healthcare_data.csv", header = TRUE)
# Clean and prepare data
df_clean <- df %>% select(Life.Expectancy, Healthcare.Spending) %>% drop_na()
df_scaled <- scale(df_clean)
# Elbow analysis
wss <- sapply(1:10, function(k){
kmeans(df_scaled, k, nstart = 10)$tot.withinss
})
plot(1:10, wss, type = "b", pch = 19, frame = FALSE,
xlab = "Number of clusters K", ylab = "Total within-clusters sum of squares")
```
I hope this helps you determine the optimal number of clusters for your k-means model!
阅读全文
相关推荐





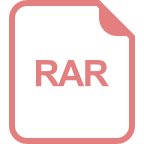










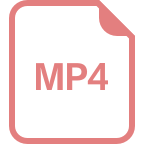