2进制转16进制代码C语言
时间: 2024-12-24 07:19:42 浏览: 3
在C语言中,将二进制数转换成十六进制数通常需要两个步骤:首先将二进制数分割成每四位一组,然后将每组转换为对应的十六进制字符。下面是一个简单的函数示例,它接受一个字符串形式的二进制数字并返回其十六进制表示:
```c
#include <stdio.h>
#include <string.h>
// Function to convert binary to hexadecimal
char* binary_to_hex(char* binary) {
int len = strlen(binary);
char hex[8]; // Assuming at most 32 bits (8 bytes), which can be represented by 2 chars for each nibble
int i = 0;
// Convert binary to hexadecimal
while (len >= 4) {
hex[i++] = '0';
hex[i++] = 'x'; // Adding '0x' prefix
int digit = 0;
for (int j = len - 4; j >= len - 8; j--) {
digit <<= 1;
if (binary[j] == '1') {
digit |= 1;
}
}
hex[i++] = digit + '0';
hex[i++] = digit > 9 ? digit - 10 + 'A' : digit + '0';
len -= 4;
}
// Handle remaining bits (if any)
if (len != 0) {
hex[i] = '0';
hex[i+1] = 'x'; // Add '0x'
int remainder = 0;
for (int j = len - 1; j >= 0; j--) {
remainder <<= 1;
if (binary[j] == '1') {
remainder |= 1;
}
}
hex[i+2] = remainder + '0';
hex[i+3] = remainder > 9 ? remainder - 10 + 'A' : remainder + '0';
}
hex[i] = '\0'; // Append null terminator
return hex;
}
int main() {
char binary[] = "1010101010101010"; // Example binary number
char* hex_result = binary_to_hex(binary);
printf("Binary %s in hexadecimal is %s\n", binary, hex_result);
free(hex_result); // Don't forget to free the dynamically allocated memory
return 0;
}
```
阅读全文
相关推荐
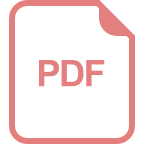
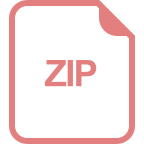
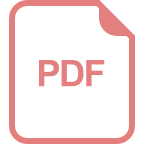















