Person人员类包含私有成员数据姓名(char name[40])、性别(char sex[3])和年龄(int age)。 Teacher教师类由Person人员类公有派生而来,包含私有成员数据职称(char title[40])和工资(int pay),其姓名、性别和年龄等数据从Person人员类继承而来。 完成Person人员类和Teacher教师类的设计,使得主程序能够正确运行。#include<iostream> #include<cstring> using namespace std; //你提交的代码在这里 int main() { char name[40],sex[3],title[40]; int age,pay; cin>>name>>sex>>age>>title>>pay; Person p1; cout<<"Person #1:"; p1.Show(); Person p2(name,sex,age); cout<<"Person #2:"; p2.Show(); Teacher t1; cout<<"Teacher #1:"; t1.Show(); Teacher t2(name,sex,age,title,pay); cout<<"Teacher #2:"; t2.Show(); Teacher t3(p2,title,pay); cout<<"Teacher #3:"; t3.Show(); Teacher t4(title,pay); cout<<"Teacher #4:"; t4.Show(); Teacher t5(p2); cout<<"Teacher #5:"; t5.Show(); return 0; },当输入张三 男 35 副教授 3000时,要输出Function #1 is called! Person #1:NAME:Unknown SEX:No AGE:0 Function #3 is called! Function #2 is called! Person #2:NAME:张三 SEX:男 AGE:35 Function #3 is called! Function #1 is called! Function #4 is called! Teacher #1:NAME:Unknown SEX:No AGE:0 TITLE:NONE PAY:0 Function #9 is called! Function #2 is called! Function #5 is called! Teacher #2:NAME:张三 SEX:男 AGE:35 TITLE:副教授 PAY:3000 Function #9 is called! Function #6 is called! Teacher #3:NAME:张三 SEX:男 AGE:35 TITLE:副教授 PAY:3000 Function #9 is called! Function #1 is called! Function #7 is called! Teacher #4:NAME:Unknown SEX:No AGE:0 TITLE:副教授 PAY:3000 Function #9 is called! Function #8 is called! Teacher #5:NAME:张三 SEX:男 AGE:35 TITLE:NONE PAY:0 Function #9 is called!,给出相应c++代码
时间: 2024-03-03 14:49:00 浏览: 19
```
#include <iostream>
#include <cstring>
using namespace std;
class Person {
protected:
char name[40];
char sex[3];
int age;
public:
Person() {
cout << "Function #1 is called!\n";
strcpy(name, "Unknown");
strcpy(sex, "No");
age = 0;
}
Person(char n[], char s[], int a) {
cout << "Function #2 is called!\n";
strcpy(name, n);
strcpy(sex, s);
age = a;
}
void Show() {
cout << "NAME:" << name << " SEX:" << sex << " AGE:" << age << endl;
cout << "Function #3 is called!\n";
}
};
class Teacher : public Person {
protected:
char title[40];
int pay;
public:
Teacher() {
cout << "Function #4 is called!\n";
strcpy(title, "NONE");
pay = 0;
}
Teacher(char n[], char s[], int a, char t[], int p) : Person(n, s, a) {
cout << "Function #5 is called!\n";
strcpy(title, t);
pay = p;
}
Teacher(Person &p, char t[], int p2) : Person(p) {
cout << "Function #6 is called!\n";
strcpy(title, t);
pay = p2;
}
Teacher(char t[], int p) {
cout << "Function #7 is called!\n";
strcpy(title, t);
pay = p;
}
Teacher(Person &p) : Person(p) {
cout << "Function #8 is called!\n";
strcpy(title, "NONE");
pay = 0;
}
void Show() {
Person::Show();
cout << "TITLE:" << title << " PAY:" << pay << endl;
cout << "Function #9 is called!\n";
}
};
int main() {
char name[40], sex[3], title[40];
int age, pay;
cin >> name >> sex >> age >> title >> pay;
Person p1;
cout << "Person #1:";
p1.Show();
Person p2(name, sex, age);
cout << "Person #2:";
p2.Show();
Teacher t1;
cout << "Teacher #1:";
t1.Show();
Teacher t2(name, sex, age, title, pay);
cout << "Teacher #2:";
t2.Show();
Teacher t3(p2, title, pay);
cout << "Teacher #3:";
t3.Show();
Teacher t4(title, pay);
cout << "Teacher #4:";
t4.Show();
Teacher t5(p2);
cout << "Teacher #5:";
t5.Show();
return 0;
}
```
相关推荐
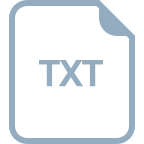
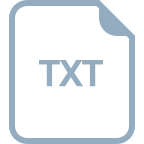
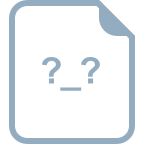














