linux qt pthread_create用法
时间: 2023-11-08 08:59:55 浏览: 95
pthread_create函数是Linux下用于创建新线程的函数,它的使用方法如下:
```c++
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void*), void *arg);
```
- thread:指向pthread_t类型的指针,用于存储新线程的标识符。
- attr:指向pthread_attr_t类型的指针,用于设置新线程的属性,通常置为NULL表示使用默认属性。
- start_routine:指向一个函数的指针,新线程将从这个函数开始执行。
- arg:传递给start_routine函数的参数。
例如,下面的示例代码演示了如何使用pthread_create创建一个新线程:
```c++
#include <iostream>
#include <pthread.h>
void* threadFunction(void* arg) {
int threadId = *(static_cast<int*>(arg));
std::cout << "Hello from thread " << threadId << std::endl;
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int threadId = 123;
int result = pthread_create(&thread, NULL, threadFunction, &threadId);
if (result == 0) {
std::cout << "Thread created successfully." << std::endl;
pthread_join(thread, NULL);
} else {
std::cout << "Failed to create thread." << std::endl;
}
return 0;
}
```
运行上述代码会创建一个新线程,该线程会打印出"Hello from thread 123"。注意,需要在主线程中调用`pthread_join`函数等待新线程结束。
相关推荐
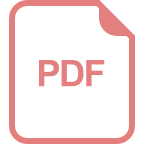
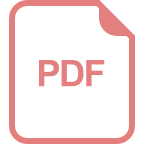














