c# Replace("0", "?").Replace("2", "0")
时间: 2024-04-05 20:27:51 浏览: 103
在C#中,Replace方法用于替换字符串中的指定字符或字符串。对于你提供的示例代码`Replace("0", "?").Replace("2", "0")`,它的作用是将字符串中的"0"替换为"?",然后将替换后的字符串中的"2"替换为"0"。
具体步骤如下:
1. 首先,调用Replace方法将字符串中的所有"0"替换为"?",得到一个新的字符串。
2. 然后,再次调用Replace方法将上一步得到的新字符串中的所有"2"替换为"0",得到最终的结果。
请注意,这两个Replace方法是按照顺序依次执行的,即先执行第一个Replace方法,再执行第二个Replace方法。
相关问题
1.Explain how bytecode (either CIL or Java bytecode) differs from machine code with examples of instructions. 2.Why autoboxing and autounboxing is not needed in C#? 3.How to decide when and when not to use auto-implemented properties in C#? 4.What is the difference between structs and class in C#? 5.How to decide between using a regular parameter declaration and a ref parameter declaration in a C# method? 6.What is the difference between modifiers new and override in C#? 7.How to decide between using a two-dimensional array and an array of arrays in a programming situation in C#? 8.Discuss the common errors that can arise with switch statements in Java and how C# eliminates those errors automatically. 9.How to use foreach loop in C#? Give one example. 10.What does this program print on the screen? Explain your answer by describing what a delegate is, including the meaning of +=. class C { delegate bool Check (int number); static bool IsEven (int number) { return number % 2 == 0;} static bool IsSmallerThan3 (int number) { return number < 3;} static void Main () { Check f = IsEven; f += IsSmallerThan3; Console.WriteLine ( f(3)); Console.WriteLine ( f(2)); } }
1. Bytecode is an intermediate code that is generated by a compiler to be executed by a virtual machine. It is platform-independent and can be executed on any platform that has a virtual machine installed. Machine code, on the other hand, is the binary code that is directly executed by a computer's CPU. Here are some examples of instructions in bytecode and machine code:
- Bytecode (CIL): ldloc.0 (load local variable 0), ldc.i4.5 (load constant value 5), add (addition)
- Machine code: mov eax, [ebp-4] (move value from memory to register), mov ebx, 5 (move constant value to register), add eax, ebx (addition)
2. Autoboxing and autounboxing is not needed in C# because C# has a feature called "value types" that allows the value types to be treated like objects. This means that value types can be used in collections and passed as parameters to methods without the need for autoboxing and autounboxing.
3. Auto-implemented properties should be used when there is no additional logic required for the getter or setter methods. If additional logic is required, then a regular property with custom getter or setter methods should be used.
4. Structs and classes are similar in that they both can contain fields, methods, and properties. The main difference is that structs are value types, while classes are reference types. This means that structs are passed by value, while classes are passed by reference.
5. A regular parameter declaration should be used when the method only needs to read the value of the parameter. A ref parameter declaration should be used when the method needs to modify the value of the parameter.
6. The "new" modifier is used to hide a method or property in a derived class, while the "override" modifier is used to replace a method or property in a base class with a new implementation in a derived class.
7. A two-dimensional array should be used when the data is logically a matrix or a table with rows and columns. An array of arrays should be used when the data is logically a collection of arrays.
8. In Java, the switch statement can cause errors if there are duplicate case values or if the break statement is not used after each case. In C#, the switch statement automatically eliminates these errors by requiring a default case and not allowing fall-through cases.
9. The foreach loop is used to iterate over a collection of items, such as an array or a list. Here is an example:
```
int[] numbers = { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
```
10. The program will print "True" and "False" because the delegate "f" contains two methods, "IsEven" and "IsSmallerThan3", which are both called when "f" is invoked with a parameter. The "+=" operator is used to add the "IsSmallerThan3" method to the delegate "f". A delegate is a type that encapsulates a method or a group of methods, and the "+=" operator is used to add a method to a delegate's invocation list.
C# replace
在C#中,`replace`是一个字符串方法,用于替换字符串中的指定字符或子字符串。它的语法如下:
```csharp
string replacedString = originalString.Replace(oldValue, newValue);
```
其中,`originalString`是要进行替换操作的原始字符串,`oldValue`是要被替换的字符或子字符串,`newValue`是替换后的新字符或子字符串。
`replace`方法会返回一个新的字符串,其中所有出现的`oldValue`都被替换为`newValue`。如果原始字符串中没有找到要替换的内容,那么返回的字符串与原始字符串相同。
需要注意的是,`replace`方法是区分大小写的。如果需要进行不区分大小写的替换操作,可以使用`StringComparison`枚举类型中的成员作为第三个参数,例如:
```csharp
string replacedString = originalString.Replace(oldValue, newValue, StringComparison.OrdinalIgnoreCase);
```
这样就会进行不区分大小写的替换操作。
阅读全文
相关推荐
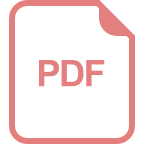
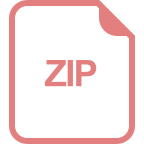
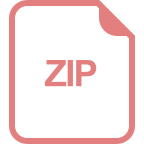
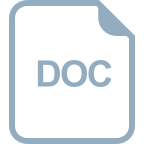
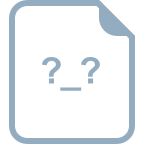
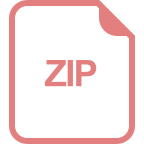
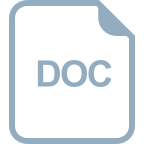
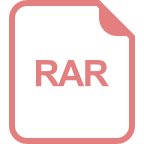
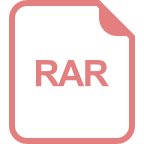
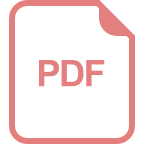


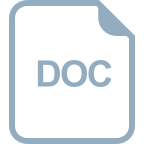
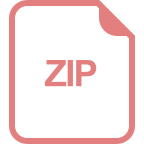
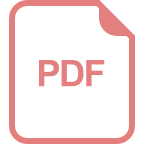
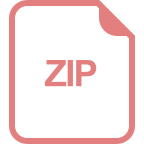