QT C++ qcustomplot 设置刻度数量
时间: 2024-12-17 20:27:29 浏览: 37
在QT C++中,使用qCustomPlot库设置图表的刻度数量,你可以通过`QCPAxisTicker`和`QCPAxis`来控制。以下是一个基本步骤:
1. 首先,你需要包含必要的头文件:
```cpp
#include <qcustomplot.h>
```
2. 创建一个轴实例,并指定你想使用的刻度计数器:
```cpp
QCPAxis *xaxis = new QCPAxis(QCPAxis::Bottom);
QCPLogTicker ticker(xaxis); // 使用对数刻度(如果你想)
ticker.setTickCount(5); // 设置为5个刻度,可以根据需要调整
xaxis->setTicker(&ticker);
```
如果你想要等距的刻度,可以使用`QCPLinearTicker`代替。
3. 将轴添加到绘图区域:
```cpp
QCPGraph *graph = new QCPGraph();
QCPPlotArea *plotArea = new QCPPlotArea;
plotArea->addAxis(xaxis);
// ... 其他配置...
graph->setAxisRect(plotArea->boundingRect());
plotArea->addGraph(graph);
```
4. 最后,在布局和显示你的窗口时,记得更新坐标系:
```cpp
ui->layout.addWidget(plotArea); // 或者你的布局容器
graph->rescaleAxes(); // 刷新刻度
```
相关问题
QT C++ QCustomPlot 设置xAxis2设置轴上一段区间的颜色及粗细
在QT C++中,使用QCustomPlot库进行定制绘图时,你可以通过设置`QCPAxis`的属性来控制xAxis2(假设它是第二条X轴)的颜色和线宽。以下是一个简单的步骤:
1. 首先,你需要包含必要的头文件:
```cpp
#include <QCustomPlot>
#include <QPen>
```
2. 然后,在初始化`QCustomPlot`实例时,创建并配置xAxis2:
```cpp
QCPAxis *xAxis2 = new QCPAxis(QCPAxis::Bottom);
plot->addAxis(xAxis2);
xAxis2->setLabel("Second X Axis");
// 创建一个自定义的轴线样式
QPen customPen(QColor("blue")); // 指定颜色,这里以蓝色为例
customPen.setWidth(2); // 设置线宽为2像素
// 定义一个区间范围
double startRange = ...; // 起始点
double endRange = ...; // 结束点
// 使用`QCPAxisTicker`选择合适的刻度
QCPAxisTicker *ticker = xAxis2->createTicker(QCPAxisTicker::Linear);
ticker->setRange(startRange, endRange);
// 将自定义的轴线风格应用到指定区间
for (double value : ticker->values()) {
if (value >= startRange && value <= endRange) {
xAxis2->setPen(value, customPen); // 设置当前值对应的轴线颜色和宽度
}
}
```
记得将起始点`startRange`和结束点`endRange`替换为你实际需要的数值。
QT C++ QCustomPlot控件 坐标刻度显示值与实际点数值是不同物理单位大小不一致,如何映射
在QT C++中的QCustomPlot控件中,如果你遇到了坐标刻度显示的值与实际数据点对应的物理单位不一致的问题,你可以通过自定义轴的标签映射函数来解决。这个函数通常会在`QCPAxisTicker::ticks()`方法中设置。以下是步骤:
1. 首先,你需要创建一个`QCPTickFormat`对象来定义新的标签格式。在这个格式中,你可以指定小数位数、单位或其他格式化信息。
```cpp
QCPTickFormat tickFormat;
tickFormat.format("%g %s"); // 格式化字符串,其中%g表示数值,%s表示单位
```
2. 接下来,针对特定的轴(例如X轴),获取当前的轴 ticker,并设置其格式:
```cpp
QCPAxis *axis = plot->xBottom; // 假设是底部X轴
axis->setTickFormat(&tickFormat);
```
3. 如果需要根据实际的数据点值动态转换单位,你可以在`ticks()`方法的回调函数中做处理。例如,当计算标签值时,可以先获取数据点值,然后将其转换为你想要的单位:
```cpp
double dataValue = yourDataValue; // 从数据源获取的实际数值
double mappedValue = convertUnit(dataValue, "original_unit", "target_unit");
axis->ticks().append(mappedValue); // 更新刻度值
```
这里,`convertUnit()`是你自己定义的一个函数,用于完成单位转换。
4. 最后,记得定期更新或同步`ticks()`以便控制面板实时反映你的更改。
阅读全文
相关推荐












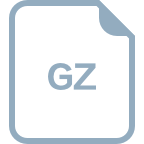



