中文np.loadtxt()
时间: 2024-06-01 08:11:45 浏览: 16
np.loadtxt()是一个numpy函数,用于从文本文件中加载数据。它返回一个numpy数组,其中包含从文件中读取的数据。
语法:np.loadtxt(fname, dtype=float, comments='#', delimiter=None, converters=None, skiprows=0, usecols=None, unpack=False, ndmin=0, encoding='bytes', max_rows=None)
参数说明:
- fname:文件名或文件的路径
- dtype:数据类型,默认为float
- comments:注释符号,默认为'#'
- delimiter:分隔符,默认为None
- converters:转换器函数,默认为None
- skiprows:要跳过的行数,默认为0
- usecols:要读取的列数,默认为None
- unpack:如果为True,则返回的数组将被转置,默认为False
- ndmin:最小维数,默认为0
- encoding:文件编码,默认为'bytes'
- max_rows:最大行数,默认为None(读取所有行)
相关问题
The above exception was the direct cause of the following exception: Traceback (most recent call last): File "C:\Users\wangchao\PycharmProjects\pythonProject\温控模型1.py", line 62, in <module> data = np.loadtxt('data.csv', delimiter=',') File "D:\anaconda\lib\site-packages\numpy\lib\npyio.py", line 1338, in loadtxt arr = _read(fname, dtype=dtype, comment=comment, delimiter=delimiter, File "D:\anaconda\lib\site-packages\numpy\lib\npyio.py", line 999, in _read arr = _load_from_filelike( ValueError: could not convert string '最高温度' to float64 at row 0, column 1.
这个错误是因为在读取 'data.csv' 文件时,第一行的第二列 '最高温度' 被尝试转换为 float64 类型,但是它不是一个数字,导致无法进行转换。你需要检查该文件的格式和内容,确保数据格式正确。如果第一行是标题行,你可以使用 `np.genfromtxt` 函数并指定跳过第一行来读取数据。例如:
```python
data = np.genfromtxt('data.csv', delimiter=',', skip_header=1)
```
如果你需要在列名中包含中文,可以使用 `pandas` 库来读取数据,它支持读取含有中文的 csv 文件,并且可以直接将列名转换为 pandas 中的列名格式。例如:
```python
import pandas as pd
data = pd.read_csv('data.csv', encoding='gbk')
```
其中 `encoding='gbk'` 是指定文件编码格式为 gbk,如果你的文件编码格式不同,可以根据实际情况进行修改。
用python写一个关于机器学习的手写汉字识别
好的,我可以为您提供一个简单的机器学习项目,用 Python 编写手写汉字识别程序。以下是基本的步骤:
1. 数据收集:收集足够多的手写汉字图片作为训练数据和测试数据。可以使用已有的数据集,例如CASIA Online and Offline Chinese Handwriting Databases,或者自己手写汉字并扫描成图片。
2. 数据处理:将收集到的图片进行处理,例如调整大小、转换成灰度图像、归一化等,以便于模型的训练和预测。
3. 特征提取:使用特征提取算法将图像中的特征提取出来,例如Hu矩、Zernike矩或SIFT特征等。
4. 模型选择和训练:选择适当的分类器(例如SVM、KNN或神经网络),使用训练数据集对分类器进行训练。在训练过程中,可以使用交叉验证等方法来调整模型参数,以提高模型的准确率。
5. 测试和评估:使用测试数据集对训练好的模型进行测试,并计算模型的准确率、召回率和F1分数等指标。
下面是一个简单的代码示例,用于手写数字识别。您可以根据需要进行修改和扩展:
```python
import numpy as np
import cv2
from sklearn import svm
from sklearn.externals import joblib
# 收集和处理数据
def load_data():
# 加载图片和标签
data = np.loadtxt('data.txt', delimiter=',')
images = np.array(data[:, :-1], dtype=np.uint8)
labels = np.array(data[:, -1], dtype=np.uint8)
return images, labels
# 提取图像特征
def extract_features(images):
# 这里使用简单的像素值作为特征
features = images.reshape(images.shape[0], -1)
return features
# 训练模型
def train_model(features, labels):
# 使用SVM作为分类器
clf = svm.SVC(kernel='linear', C=1.0)
clf.fit(features, labels)
return clf
# 测试模型
def test_model(clf, test_images, test_labels):
# 对测试集进行预测
predictions = clf.predict(test_images)
# 计算准确率
accuracy = np.mean(predictions == test_labels) * 100
print('Accuracy:', accuracy)
# 加载数据
images, labels = load_data()
# 划分训练集和测试集
train_images, train_labels = images[:5000], labels[:5000]
test_images, test_labels = images[5000:], labels[5000:]
# 提取特征
train_features = extract_features(train_images)
test_features = extract_features(test_images)
# 训练模型
clf = train_model(train_features, train_labels)
# 测试模型
test_model(clf, test_features, test_labels)
# 保存模型
joblib.dump(clf, 'model.pkl')
```
在上述示例中,我们使用SVM作为分类器,使用像素值作为特征,并将训练好的模型保存到文件中。您可以根据需要修改这些参数和方法,以适应您的数据和需求。
相关推荐
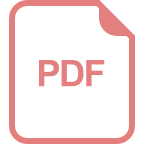
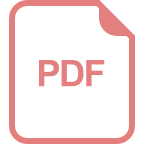







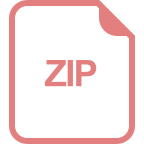
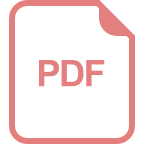
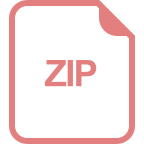
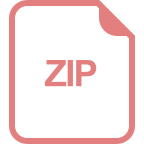