testng接口自动化测试
时间: 2023-09-24 21:06:20 浏览: 159
TestNG是一个基于Java的测试框架,它可以帮助我们进行单元测试、集成测试、功能测试等各种类型的测试。TestNG旨在提供更好的灵活性和更丰富的功能,以及更好的报告和日志记录。TestNG是JUnit的一个更好的替代方案,它的一些优势包括:
- TestNG支持注释来进行配置测试,这使得测试更加灵活和易于管理。
- TestNG支持测试套件和测试组,这使得测试更容易组织和管理。
- TestNG支持并发执行测试,这可以节省测试时间并提高效率。
- TestNG提供了更好的测试报告和日志记录功能,这有助于更好地理解测试结果。
在使用TestNG进行接口自动化测试时,我们可以使用TestNG提供的注释来标记测试方法、测试类、测试套件等,以便更好地组织测试。我们可以使用TestNG提供的断言来验证接口返回结果是否符合预期,还可以使用TestNG提供的数据提供者来对接口进行多组数据测试。
接下来是一个使用TestNG进行接口自动化测试的示例:
```java
import org.testng.Assert;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
public class ApiTest {
@Test(dataProvider = "testdata")
public void testApi(String url, String method, String requestBody, int expectedStatusCode) {
RequestSpecification request = RestAssured.given().body(requestBody);
Response response = null;
if (method.equals("GET")) {
response = request.get(url);
} else if (method.equals("POST")) {
response = request.post(url);
} else if (method.equals("PUT")) {
response = request.put(url);
} else if (method.equals("DELETE")) {
response = request.delete(url);
}
Assert.assertEquals(response.getStatusCode(), expectedStatusCode);
}
@DataProvider(name = "testdata")
public Object[][] testData() {
Object[][] data = new Object[][] {
{"https://example.com/api/users/1", "GET", null, 200},
{"https://example.com/api/users", "POST", "{\"name\":\"John\",\"age\":30}", 201},
{"https://example.com/api/users/1", "PUT", "{\"name\":\"John Doe\",\"age\":32}", 200},
{"https://example.com/api/users/1", "DELETE", null, 204}
};
return data;
}
}
```
在这个示例中,我们使用了RestAssured库来发送HTTP请求并获取响应。在testApi方法中,我们根据传入的参数选择不同的HTTP方法,并发送请求。然后,我们使用TestNG的断言来验证响应状态码是否符合预期。在testData数据提供者中,我们定义了一组测试数据,每组数据包括接口URL、HTTP方法、请求体和预期状态码。测试方法会对每组数据进行测试。
总之,使用TestNG进行接口自动化测试可以让我们更好地组织和管理测试,提高测试效率和可靠性。
阅读全文
相关推荐
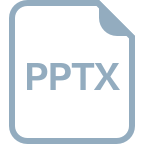
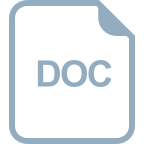
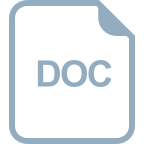



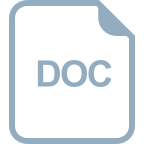




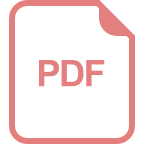
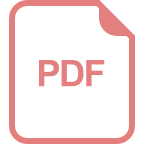
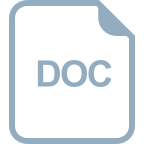
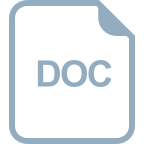
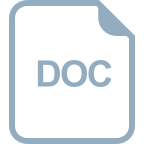