快速排序 用C语言
时间: 2023-11-24 08:52:33 浏览: 100
以下是使用C语言实现快速排序的代码:
```c
#include <stdio.h>
// 单趟排序
int PartSort(int* arr, int begin, int end) {
int key = arr[end];
while (begin < end) {
while (begin < end && arr[begin] <= key) {
++begin;
}
arr[end] = arr[begin];
while (begin < end && arr[end] >= key) {
--end;
}
arr[begin] = arr[end];
}
arr[end] = key;
return end;
}
// 快速排序递归实现
void QuickSort(int* arr, int begin, int end) {
if (begin >= end) {
return;
}
int key = PartSort(arr, begin, end);
QuickSort(arr, begin, key - 1);
QuickSort(arr, key + 1, end);
}
// 快速排序非递归实现
void QuickSortNonRecursive(int* arr, int begin, int end) {
int stack[end - begin + 1];
int top = -1;
stack[++top] = begin;
stack[++top] = end;
while (top >= 0) {
int right = stack[top--];
int left = stack[top--];
int key = PartSort(arr, left, right);
if (key - 1 > left) {
stack[++top] = left;
stack[++top] = key - 1;
}
if (key + 1 < right) {
stack[++top] = key + 1;
stack[++top] = right;
}
}
}
int main() {
int arr[] = { 5, 3, 8, 4, 2, 7, 1, 6 };
int len = sizeof(arr) / sizeof(arr[0]);
QuickSort(arr, 0, len - 1);
for (int i = 0; i < len; ++i) {
printf("%d ", arr[i]);
}
printf("\n");
QuickSortNonRecursive(arr, 0, len - 1);
for (int i = 0; i < len; ++i) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐


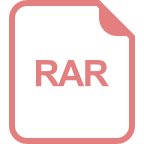

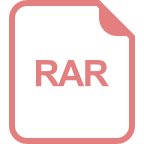

