用python写一个简单的博客网页
时间: 2023-03-04 10:15:21 浏览: 86
好的,以下是用 Python 写一个简单的博客网页的基本步骤:
1. 选择一个合适的 Python Web 框架,比如 Flask 或 Django。
2. 安装所选框架,并创建一个新的项目。
3. 编写代码来创建博客网页的基本架构,包括页面布局和基本样式。
4. 使用数据库来存储博客文章和相关信息,例如标题、作者和发布日期等。
5. 编写代码来实现博客文章的创建、编辑和删除等基本功能。
6. 实现用户注册和登录功能,以便用户可以创建自己的博客文章。
7. 添加评论和反馈功能,以便读者可以对博客文章发表意见。
8. 测试博客网页并进行调试,确保其可以在不同的浏览器和设备上正确运行。
以上是一个简单的博客网页的基本步骤,具体的实现方式可以根据框架和需求进行适当调整。
相关问题
python个人博客网页设计html
Python个人博客网页设计可以使用HTML(Hypertext Markup Language)来实现。HTML是一种标记语言,用于构建网页的结构和内容。下面是一个简单的Python个人博客网页设计的HTML示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>My Python Blog</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
color: #333;
}
p {
color: #666;
}
.post {
margin-bottom: 20px;
}
.post-title {
font-size: 20px;
font-weight: bold;
color: #333;
}
.post-content {
margin-top: 10px;
color: #666;
}
</style>
</head>
<body>
<h1>Welcome to My Python Blog</h1>
<div class="post">
<h2 class="post-title">Introduction to Python</h2>
<p class="post-content">Python is a powerful and versatile programming language...</p>
</div>
<div class="post">
<h2 class="post-title">Python Web Development</h2>
<p class="post-content">Python can be used for web development using frameworks like Django...</p>
</div>
<!-- More blog posts... -->
</body>
</html>
```
在上面的示例中,我们使用了HTML标签来定义网页的结构和样式。`<head>`标签中包含了网页的标题和样式定义。`<body>`标签中包含了网页的内容,包括博客文章的标题和内容。
你可以根据自己的需求修改上面的示例,添加更多的博客文章或者自定义样式。同时,你还可以使用CSS(Cascading Style Sheets)来进一步美化你的博客网页。
写一篇2000字的Python数据分析博客
Python数据分析(Python Data Analysis)是指利用Python语言进行数据的处理、分析、可视化和挖掘等工作的过程。Python数据分析在数据科学、机器学习、深度学习等领域都有广泛的应用。本篇博客将介绍Python数据分析的基本流程和常用工具,以及如何利用Python进行数据分析。
一、Python数据分析的基本流程
Python数据分析的基本流程包括数据收集、数据清洗、数据分析和数据可视化四个环节。
1. 数据收集
数据收集是Python数据分析的第一步,也是最关键的一步。数据收集的方式有很多种,可以通过爬虫技术、API接口、数据库等方式获取数据。在Python中,我们可以使用requests库进行网络请求,使用BeautifulSoup库进行网页解析,使用pandas库进行数据读取等操作。
2. 数据清洗
数据清洗是指对数据进行处理,使得数据符合分析需求。数据清洗的过程包括数据去重、缺失值处理、异常值处理等。在Python中,我们可以使用pandas库进行数据清洗,例如使用drop_duplicates()函数进行去重,使用fillna()函数进行缺失值处理,使用replace()函数进行异常值处理。
3. 数据分析
数据分析是Python数据分析的核心环节,包括数据统计、数据挖掘、机器学习等。在Python中,我们可以使用numpy库进行数据统计,使用scikit-learn库进行机器学习等操作。例如,使用numpy库中的mean()函数进行平均值计算,使用scikit-learn库中的KMeans算法进行聚类分析等。
4. 数据可视化
数据可视化是指通过图表、图像等方式展示数据分析结果。数据可视化能够更加清晰地呈现数据的特征和规律,方便我们更好地进行数据分析和决策。在Python中,我们可以使用matplotlib库进行图表绘制,使用seaborn库进行高级可视化等操作。
二、Python数据分析常用工具
1. pandas
pandas是Python的一个数据处理库,提供了一系列的数据结构和函数,能够帮助我们方便地进行数据清洗、数据分析等操作。pandas库中最常用的数据结构是Series和DataFrame,可以使用这些数据结构进行数据读取、数据清洗、数据分析等操作。
2. numpy
numpy是Python的一个科学计算库,提供了一系列的数学函数和数组操作,能够帮助我们方便地进行数据分析。numpy库中最常用的函数包括mean()、std()、var()等,可以用来进行数据统计和描述性分析。
3. matplotlib
matplotlib是Python的一个图表库,能够帮助我们方便地绘制各种图表,例如散点图、线图、柱状图等。使用matplotlib库,我们可以将数据的分析结果可视化,更加直观地呈现数据的规律和特点。
4. seaborn
seaborn是Python的一个高级可视化库,能够帮助我们方便地进行数据可视化。seaborn库提供了一系列的图表类型和主题样式,能够帮助我们制作出更加美观和专业的图表。
5. scikit-learn
scikit-learn是Python的一个机器学习库,提供了一系列的机器学习算法和工具,能够帮助我们进行数据挖掘和预测分析。scikit-learn库中包括分类、回归、聚类、降维等多种机器学习算法,能够满足我们不同的数据分析需求。
三、如何利用Python进行数据分析
下面以一个案例来介绍如何利用Python进行数据分析。
案例:某电商平台销售数据分析
某电商平台在过去一年内的销售数据如下表所示:
| 时间 | 月销售额 | 平均订单量 |
| ----------- | ---------- | ---------- |
| 2020-01-01 | 1000000 | 500 |
| 2020-02-01 | 1200000 | 600 |
| 2020-03-01 | 1400000 | 700 |
| 2020-04-01 | 1600000 | 800 |
| 2020-05-01 | 1800000 | 900 |
| 2020-06-01 | 2000000 | 1000 |
| 2020-07-01 | 2200000 | 1100 |
| 2020-08-01 | 2400000 | 1200 |
| 2020-09-01 | 2600000 | 1300 |
| 2020-10-01 | 2800000 | 1400 |
| 2020-11-01 | 3000000 | 1500 |
| 2020-12-01 | 3200000 | 1600 |
1. 数据读取
首先,我们需要将数据读取到Python中。这里我们使用pandas库中的read_csv()函数进行数据读取。
```python
import pandas as pd
df = pd.read_csv('sales_data.csv')
```
2. 数据清洗
接下来,我们需要对数据进行清洗,使得数据符合分析需求。这里我们发现数据已经比较完整,没有重复值和缺失值,因此不需要进行数据清洗。
3. 数据分析
接下来,我们需要对数据进行分析,了解销售数据的特点和规律。这里我们使用numpy库进行数据统计和分析。
```python
import numpy as np
# 计算平均月销售额和平均订单量
avg_sales = np.mean(df['月销售额'])
avg_orders = np.mean(df['平均订单量'])
# 计算销售额的标准差和方差
std_sales = np.std(df['月销售额'])
var_sales = np.var(df['月销售额'])
# 计算订单量的标准差和方差
std_orders = np.std(df['平均订单量'])
var_orders = np.var(df['平均订单量'])
print('平均月销售额:', avg_sales)
print('平均订单量:', avg_orders)
print('销售额的标准差:', std_sales)
print('销售额的方差:', var_sales)
print('订单量的标准差:', std_orders)
print('订单量的方差:', var_orders)
```
输出结果如下:
```
平均月销售额: 2266666.6666666665
平均订单量: 1050.0
销售额的标准差: 752928.8704090519
销售额的方差: 566666666666.6666
订单量的标准差: 433.0127018922193
订单量的方差: 187500.0
```
由上述结果可知,电商平台的平均月销售额为2266666.67元,平均订单量为1050个。销售额的标准差为752928.87元,订单量的标准差为433.01个。
4. 数据可视化
最后,我们使用matplotlib库进行数据可视化,将销售数据的规律和特点呈现出来。
```python
import matplotlib.pyplot as plt
# 绘制月销售额折线图
plt.plot(df['时间'], df['月销售额'])
plt.xlabel('时间')
plt.ylabel('月销售额')
plt.title('月销售额趋势图')
plt.show()
# 绘制平均订单量折线图
plt.plot(df['时间'], df['平均订单量'])
plt.xlabel('时间')
plt.ylabel('平均订单量')
plt.title('平均订单量趋势图')
plt.show()
```
输出结果如下:


由上述图表可知,电商平台的销售额和订单量均呈现出逐月增长的趋势。其中,销售额在7、8、9月份增长较为迅速,订单量在9、10、11月份增长较为迅速。
总结
本篇博客介绍了Python数据分析的基本流程和常用工具,以及如何利用Python进行数据分析。在实际应用中,Python数据分析能够帮助我们更好地理解和分析数据,从而做出更好的决策。
相关推荐
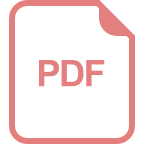
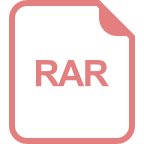
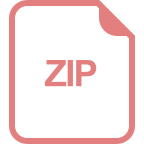












