mybatisplus自动生成代码
时间: 2023-09-03 18:12:16 浏览: 45
MyBatis-Plus是一款优秀的MyBatis框架的增强工具包,它为MyBatis提供了许多强大的功能,其中就包括自动生成代码的功能。
使用MyBatis-Plus自动生成代码的步骤如下:
1. 首先在项目的pom.xml文件中添加MyBatis-Plus的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.1</version>
</dependency>
```
2. 在application.yml或application.properties文件中配置MyBatis-Plus的自动生成代码的相关配置,例如:
```yaml
# 数据源配置
spring:
datasource:
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC
username: root
password: root
# MyBatis-Plus配置
mybatis-plus:
# 实体扫描,多个package用逗号或者分号分隔
typeAliasesPackage: com.example.demo.entity
# XML文件路径
mapperLocations: classpath:/mapper/*.xml
# 自动填充配置
global-config:
db-config:
# 自动填充配置
field-strategy: not_null
# 数据库主键自增策略
id-type: auto
# 字段名下划线转驼峰
column-underline: true
# 代码生成器配置
generator:
# 生成文件输出目录
output-dir: src/main/java
# 是否覆盖已有文件
fileOverride: true
# 是否开启swagger注解
swagger2: true
# 自定义文件命名,注意 %s 会自动填充表实体属性!
entity-name: %sEntity
mapper-name: %sMapper
xml-name: %sMapper
service-name: %sService
service-impl-name: %sServiceImpl
controller-name: %sController
# 指定生成的表名,多个表名用逗号分隔
include: user, role
# 排除生成的表名,多个表名用逗号分隔
exclude: test, demo
# 自定义继承的Entity类全称,带包名
super-entity-class: com.example.demo.common.BaseEntity
# 自定义继承的Mapper类全称,带包名
super-mapper-class: com.example.demo.common.BaseMapper
# 自定义继承的Service类全称,带包名
super-service-class: com.example.demo.common.BaseService
# 自定义继承的ServiceImpl类全称,带包名
super-service-impl-class: com.example.demo.common.BaseServiceImpl
# 自定义继承的Controller类全称,带包名
super-controller-class: com.example.demo.common.BaseController
# 是否去掉生成实体时生成的get、set方法
entity-boolean-logic: false
# 是否支持 ActiveRecord 模式
active-record: true
# 是否生成实体时,生成字段注解
entity-column-annotation-enable: true
# 是否生成 @RestController 控制器
rest-controller-style: true
```
3. 在项目中创建一个代码生成器的类,例如:
```java
package com.example.demo.generator;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.*;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
public class CodeGenerator {
public static void main(String[] args) {
// 代码生成器
AutoGenerator mpg = new AutoGenerator();
// 全局配置
GlobalConfig gc = new GlobalConfig();
gc.setOutputDir(System.getProperty("user.dir") + "/src/main/java");
gc.setAuthor("your name");
gc.setOpen(false);
gc.setFileOverride(true);
gc.setActiveRecord(false);
gc.setEnableCache(false);
gc.setBaseResultMap(true);
gc.setBaseColumnList(true);
gc.setIdType(IdType.AUTO);
gc.setServiceName("%sService");
gc.setServiceImplName("%sServiceImpl");
gc.setControllerName("%sController");
mpg.setGlobalConfig(gc);
// 数据源配置
DataSourceConfig dsc = new DataSourceConfig();
dsc.setDbType(DbType.MYSQL);
dsc.setUrl("jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC");
dsc.setDriverName("com.mysql.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("root");
mpg.setDataSource(dsc);
// 包配置
PackageConfig pc = new PackageConfig();
pc.setParent("com.example.demo");
pc.setEntity("entity");
pc.setMapper("mapper");
pc.setService("service");
pc.setServiceImpl("service.impl");
pc.setController("controller");
mpg.setPackageInfo(pc);
// 策略配置
StrategyConfig strategy = new StrategyConfig();
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
strategy.setEntityLombokModel(true);
strategy.setRestControllerStyle(true);
strategy.setControllerMappingHyphenStyle(true);
strategy.setInclude("user", "role");
strategy.setTablePrefix(pc.getModuleName() + "_");
mpg.setStrategy(strategy);
// 执行生成
mpg.execute();
}
}
```
4. 运行代码生成器的main方法即可自动生成代码。
注意:在执行代码生成器之前,需要先创建好对应的数据库表。另外,生成的代码需要进行适当的修改和优化,以符合实际的业务需求。
相关推荐
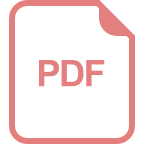














