用python写一个简单好玩的小游戏,要有界面
时间: 2024-03-06 09:49:11 浏览: 37
好,我可以为你提供一个基于 Python Tkinter 模块的简单游戏示例,名为“打地鼠游戏”:
```
import tkinter as tk
import random
# 创建主窗口
root = tk.Tk()
root.title("打地鼠游戏")
# 设置窗口大小
WIDTH = 400
HEIGHT = 400
root.geometry(f"{WIDTH}x{HEIGHT}")
# 创建游戏区域
canvas = tk.Canvas(root, width=WIDTH, height=HEIGHT)
canvas.pack()
# 定义地鼠类
class Mole:
def __init__(self):
self.x = random.randint(50, WIDTH-50)
self.y = random.randint(50, HEIGHT-50)
self.radius = 30
self.color = "brown"
self.speed = random.randint(1000, 2000)
self.draw()
def draw(self):
self.shape = canvas.create_oval(self.x-self.radius, self.y-self.radius,
self.x+self.radius, self.y+self.radius,
fill=self.color)
def hide(self):
canvas.delete(self.shape)
# 定义游戏函数
def start_game():
global score, moles
score = 0
moles = []
for i in range(10):
moles.append(Mole())
update_score()
root.after(30000, end_game)
def end_game():
global score, moles
for mole in moles:
mole.hide()
canvas.create_text(WIDTH/2, HEIGHT/2, text=f"游戏结束!得分:{score}", font=("Arial", 24))
def update_score():
canvas.delete("score")
canvas.create_text(50, 20, text=f"得分:{score}", font=("Arial", 16), anchor="nw", tag="score")
def hit(event):
global score, moles
for mole in moles:
if abs(event.x-mole.x) < mole.radius and abs(event.y-mole.y) < mole.radius:
score += 10
update_score()
mole.hide()
moles.remove(mole)
moles.append(Mole())
# 绑定事件
canvas.bind("<Button-1>", hit)
# 创建开始按钮
button = tk.Button(root, text="开始游戏", command=start_game, font=("Arial", 20))
canvas.create_window(WIDTH/2, HEIGHT/2, window=button)
# 运行主程序
root.mainloop()
```
这个游戏创建一个主窗口,包含一个游戏区域和一个“开始游戏”按钮。游戏区域随机生成 10 个地鼠,玩家需要在 30 秒内点击地鼠来得分。当时间到或者玩家点击了所有地鼠后,游戏结束,显示得分。你可以根据自己的需求进行修改和扩展,例如增加难度、音效等等。
相关推荐
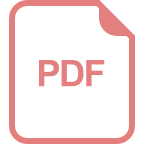
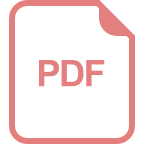














