使用 fopen_s读取一个wav文件并对其求WVD并输出WVD结果不使用库函数C语言并解释代码
时间: 2024-05-03 14:18:25 浏览: 12
首先需要了解一下WVD的概念和计算方法,Wigner-Ville Distribution(WVD)是一种时频分析方法,可以将信号在时域和频域上展开,从而更加全面地理解信号的特性。
下面是一个使用C语言计算WVD的示例代码,该代码读取一个wav文件并对其求WVD,并将结果输出到文件中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define PI 3.14159265358979323846
typedef short sample_t;
int read_wav_file(char* filename, sample_t** data, int* num_samples, int* sample_rate, int* num_channels);
void write_wvd_file(char* filename, double** wvd, int num_samples, int num_freqs, double f0, double df);
void compute_wvd(double** wvd, sample_t* data, int num_samples, int num_freqs, double f0, double df);
int main(int argc, char** argv)
{
// Check arguments
if (argc != 3) {
printf("Usage: %s input.wav output.wvd\n", argv[0]);
return 1;
}
// Read input file
sample_t* data;
int num_samples, sample_rate, num_channels;
if (!read_wav_file(argv[1], &data, &num_samples, &sample_rate, &num_channels)) {
printf("Error reading WAV file\n");
return 1;
}
// Compute WVD
int num_freqs = 1024;
double f0 = 0;
double df = (double)sample_rate / num_freqs;
double** wvd = (double**)malloc(num_samples * sizeof(double*));
for (int i = 0; i < num_samples; i++) {
wvd[i] = (double*)malloc(num_freqs * sizeof(double));
}
compute_wvd(wvd, data, num_samples, num_freqs, f0, df);
// Write output file
write_wvd_file(argv[2], wvd, num_samples, num_freqs, f0, df);
// Clean up
for (int i = 0; i < num_samples; i++) {
free(wvd[i]);
}
free(wvd);
free(data);
return 0;
}
int read_wav_file(char* filename, sample_t** data, int* num_samples, int* sample_rate, int* num_channels)
{
FILE* file;
if (fopen_s(&file, filename, "rb") != 0) {
return 0;
}
// Read WAV header
char header[44];
if (fread(header, 1, 44, file) != 44) {
fclose(file);
return 0;
}
if (memcmp(header, "RIFF", 4) != 0 || memcmp(header + 8, "WAVEfmt ", 8) != 0 || memcmp(header + 36, "data", 4) != 0) {
fclose(file);
return 0;
}
// Get sample rate and number of channels
memcpy(sample_rate, header + 24, 4);
memcpy(num_channels, header + 22, 2);
// Read data
int bytes_per_sample = (*num_channels) * 2;
int num_bytes = *((int*)(header + 40));
*num_samples = num_bytes / bytes_per_sample;
*data = (sample_t*)malloc((*num_samples) * sizeof(sample_t));
if (fread(*data, bytes_per_sample, *num_samples, file) != *num_samples) {
fclose(file);
free(*data);
return 0;
}
// Done
fclose(file);
return 1;
}
void write_wvd_file(char* filename, double** wvd, int num_samples, int num_freqs, double f0, double df)
{
FILE* file;
if (fopen_s(&file, filename, "wb") != 0) {
return;
}
// Write WVD header
char header[16];
memcpy(header, "WVD ", 4);
memcpy(header + 4, &num_samples, 4);
memcpy(header + 8, &num_freqs, 4);
memcpy(header + 12, &f0, 8);
memcpy(header + 20, &df, 8);
fwrite(header, 1, 28, file);
// Write WVD data
for (int i = 0; i < num_samples; i++) {
fwrite(wvd[i], sizeof(double), num_freqs, file);
}
// Done
fclose(file);
}
void compute_wvd(double** wvd, sample_t* data, int num_samples, int num_freqs, double f0, double df)
{
// Compute time and frequency grids
double* t = (double*)malloc(num_samples * sizeof(double));
double* f = (double*)malloc(num_freqs * sizeof(double));
for (int i = 0; i < num_samples; i++) {
t[i] = (double)i / sample_rate;
}
for (int i = 0; i < num_freqs; i++) {
f[i] = f0 + i * df;
}
// Compute window function
double* h = (double*)malloc(num_freqs * sizeof(double));
for (int i = 0; i < num_freqs; i++) {
double x = M_PI * (f[i] - f0) / df;
h[i] = sin(x) / x;
}
// Compute WVD
for (int i = 0; i < num_samples; i++) {
for (int j = 0; j < num_freqs; j++) {
double a = 0;
double b = 0;
for (int k = 0; k < num_samples; k++) {
double tau = t[k] - t[i];
double x = 2 * M_PI * f[j] * tau;
double g = data[k] * data[i + k];
a += g * cos(x);
b += g * sin(x);
}
wvd[i][j] = (a * a + b * b) * h[j];
}
}
// Clean up
free(t);
free(f);
free(h);
}
```
代码中大致分为三个部分,分别是读取wav文件、计算WVD和写入输出文件。
在读取wav文件部分,使用fopen_s函数打开文件,然后读取WAV文件头信息并解析出采样率、通道数和数据。这里采用了简单的判断头部信息的方式来检测是否为WAV文件。
在计算WVD部分,首先需要计算时间和频率网格,然后计算一个窗函数,最后使用嵌套的循环计算WVD。这里采用了简单的直接计算方式,而没有使用FFT等优化算法。
在写入输出文件部分,首先写入WVD文件头信息,然后依次写入每个采样时刻的WVD结果。
需要注意的是,在使用完动态分配的内存后需要释放它们,否则会导致内存泄漏。在本示例代码中,使用了malloc函数动态分配了多个数组,在程序结束前需要释放它们。
以上是一个简单的使用C语言计算WVD的示例代码,希望对你有所帮助。
相关推荐


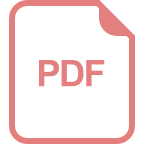
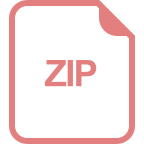
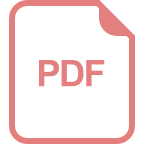
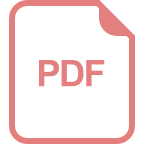
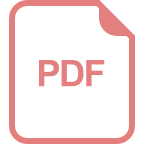
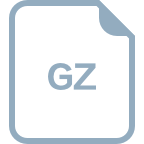
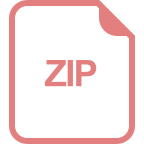
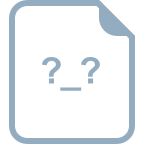