STM32语音播放模块代码
时间: 2024-06-08 22:11:12 浏览: 112
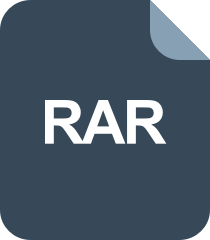
STM32_ISD1760语音播放——地址.rar_stm32_stm32 ISD1760_stm32语音_语音播放模块ISD1

以下是使用STM32F4开发板和DFPlayer Mini模块播放音频文件的示例代码:
```c
#include "stm32f4xx.h"
#include "stm32f4_discovery.h"
// UART communication with DFPlayer Mini
#define DFPLAYER_UART USART2
// Pins connected to DFPlayer Mini
#define DFPLAYER_TX GPIO_Pin_2
#define DFPLAYER_RX GPIO_Pin_3
// Commands for DFPlayer Mini
#define DFPLAYER_PLAY_NEXT 0x01
#define DFPLAYER_PLAY_PREV 0x02
#define DFPLAYER_PLAY_SPECIFIC 0x03
#define DFPLAYER_VOLUME_UP 0x04
#define DFPLAYER_VOLUME_DOWN 0x05
#define DFPLAYER_SET_VOLUME 0x06
#define DFPLAYER_SET_EQ 0x07
#define DFPLAYER_LOOP_PLAYBACK 0x08
#define DFPLAYER_STOP_PLAYBACK 0x0E
// Volume levels
#define DFPLAYER_MIN_VOLUME 0x00
#define DFPLAYER_MAX_VOLUME 0x1E
USART_InitTypeDef USART_InitStruct;
void DFPlayer_SendCommand(uint8_t command, uint16_t parameter)
{
uint8_t checksum = -(0xAA + 0x01 + 0x00 + command + (parameter >> 8) + (parameter & 0xFF));
USART_SendData(DFPLAYER_UART, 0xAA);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, 0x01);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, 0x00);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, command);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, parameter >> 8);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, parameter & 0xFF);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
USART_SendData(DFPLAYER_UART, checksum);
while (USART_GetFlagStatus(DFPLAYER_UART, USART_FLAG_TXE) == RESET);
}
void DFPlayer_PlayNext()
{
DFPlayer_SendCommand(DFPLAYER_PLAY_NEXT, 0);
}
void DFPlayer_PlayPrev()
{
DFPlayer_SendCommand(DFPLAYER_PLAY_PREV, 0);
}
void DFPlayer_PlaySpecific(uint16_t track)
{
DFPlayer_SendCommand(DFPLAYER_PLAY_SPECIFIC, track);
}
void DFPlayer_VolumeUp()
{
DFPlayer_SendCommand(DFPLAYER_VOLUME_UP, 0);
}
void DFPlayer_VolumeDown()
{
DFPlayer_SendCommand(DFPLAYER_VOLUME_DOWN, 0);
}
void DFPlayer_SetVolume(uint8_t volume)
{
if (volume > DFPLAYER_MAX_VOLUME)
{
volume = DFPLAYER_MAX_VOLUME;
}
DFPlayer_SendCommand(DFPLAYER_SET_VOLUME, volume);
}
void DFPlayer_SetEQ(uint8_t eq)
{
DFPlayer_SendCommand(DFPLAYER_SET_EQ, eq);
}
void DFPlayer_LoopPlayback()
{
DFPlayer_SendCommand(DFPLAYER_LOOP_PLAYBACK, 0);
}
void DFPlayer_StopPlayback()
{
DFPlayer_SendCommand(DFPLAYER_STOP_PLAYBACK, 0);
}
int main(void)
{
// Initialize GPIO and USART2
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = DFPLAYER_TX | DFPLAYER_RX;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_USART2);
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(DFPLAYER_UART, &USART_InitStruct);
USART_Cmd(DFPLAYER_UART, ENABLE);
// Play audio files
while (1)
{
DFPlayer_PlaySpecific(1); // Play track 1
while (1); // Wait for playback to finish
DFPlayer_PlayNext(); // Play next track
while (1); // Wait for playback to finish
}
}
```
此示例代码使用USART2与DFPlayer Mini模块进行通信,通过发送命令和参数的组合来控制音频播放。您需要根据您的硬件连接和DFPlayer Mini模块的手册来调整代码。
阅读全文
相关推荐
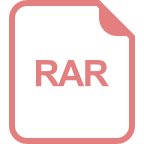


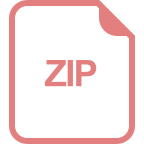
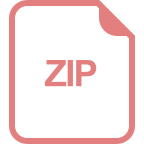
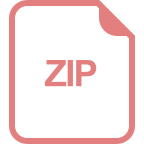









