使用axios库异步读取存储在json文件中的学生信息,绑定学生信息至表格,并为表头复选框和数据行复选框添加监听器,实现全选和选中行功能。
时间: 2024-03-08 09:51:16 浏览: 118
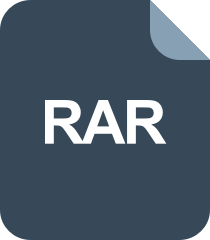
Ajax做的学生信息管理系统

以下是使用axios库异步读取存储在json文件中的学生信息,绑定学生信息至表格,并为表头复选框和数据行复选框添加监听器,实现全选和选中行功能的示例代码:
HTML代码:
```
<table>
<thead>
<tr>
<th><input type="checkbox" id="selectAll"></th>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
</tr>
</thead>
<tbody id="tableBody"></tbody>
</table>
```
JavaScript代码:
```
// 获取表格的元素和全选复选框的元素
const tableBody = document.getElementById('tableBody')
const selectAllCheckbox = document.getElementById('selectAll')
// 使用axios库异步读取存储在json文件中的学生信息
axios.get('/students.json')
.then(response => {
const students = response.data
// 将学生信息绑定至表格中
for (let i = 0; i < students.length; i++) {
const student = students[i]
const tr = document.createElement('tr')
const checkbox = document.createElement('input')
checkbox.type = 'checkbox'
checkbox.value = student.id
const td1 = document.createElement('td')
td1.appendChild(checkbox)
const td2 = document.createElement('td')
td2.innerText = student.id
const td3 = document.createElement('td')
td3.innerText = student.name
const td4 = document.createElement('td')
td4.innerText = student.gender
const td5 = document.createElement('td')
td5.innerText = student.age
tr.appendChild(td1)
tr.appendChild(td2)
tr.appendChild(td3)
tr.appendChild(td4)
tr.appendChild(td5)
tableBody.appendChild(tr)
}
// 添加全选复选框的监听器
selectAllCheckbox.addEventListener('change', () => {
const checkboxes = document.querySelectorAll('tbody input[type="checkbox"]')
for (let i = 0; i < checkboxes.length; i++) {
checkboxes[i].checked = selectAllCheckbox.checked
}
})
// 添加数据行复选框的监听器
const dataCheckboxes = document.querySelectorAll('tbody input[type="checkbox"]')
for (let i = 0; i < dataCheckboxes.length; i++) {
dataCheckboxes[i].addEventListener('change', () => {
let allChecked = true
for (let j = 0; j < dataCheckboxes.length; j++) {
if (!dataCheckboxes[j].checked) {
allChecked = false
break
}
}
selectAllCheckbox.checked = allChecked
})
}
})
.catch(error => {
console.log(error)
})
```
上述代码使用了axios库异步读取存储在json文件中的学生信息,并将学生信息绑定至表格中。同时,为表头复选框和数据行复选框添加了监听器,实现了全选和选中行的功能。
阅读全文
相关推荐
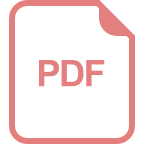
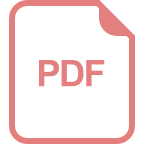
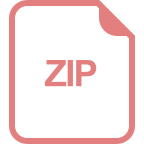
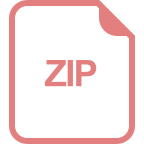
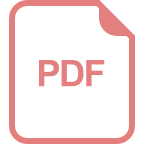
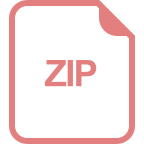
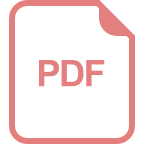
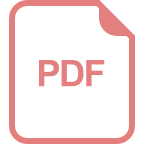
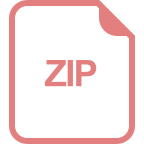
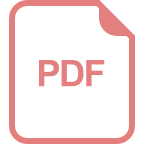
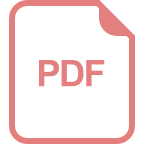
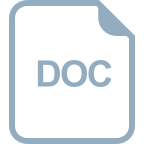
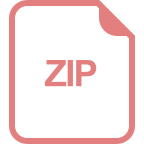