Java设计华氏温度摄氏温度相互转化的可视化界面
时间: 2023-08-16 09:04:33 浏览: 199
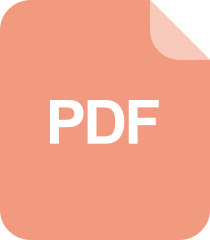
用Eclipse进行可视化Java界面设计
以下是一个简单的Java Swing界面,用于将华氏温度转换为摄氏温度,或将摄氏温度转换为华氏温度。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class TemperatureConverter extends JFrame implements ActionListener {
private JTextField inputField, outputField;
private JRadioButton fahrenheitButton, celsiusButton;
public TemperatureConverter() {
// 创建界面
setTitle("温度转换器");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(3, 2));
// 创建输入框和标签
JLabel inputLabel = new JLabel("输入温度:");
inputField = new JTextField("");
add(inputLabel);
add(inputField);
// 创建输出框和标签
JLabel outputLabel = new JLabel("转换结果:");
outputField = new JTextField("");
outputField.setEditable(false);
add(outputLabel);
add(outputField);
// 创建单选按钮组
ButtonGroup group = new ButtonGroup();
fahrenheitButton = new JRadioButton("华氏度");
celsiusButton = new JRadioButton("摄氏度");
fahrenheitButton.setSelected(true);
group.add(fahrenheitButton);
group.add(celsiusButton);
add(fahrenheitButton);
add(celsiusButton);
// 创建转换按钮
JButton convertButton = new JButton("转换");
convertButton.addActionListener(this);
add(convertButton);
// 显示界面
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
// 获取输入的温度值
String inputText = inputField.getText();
if (inputText.isEmpty()) {
JOptionPane.showMessageDialog(this, "请输入温度值");
return;
}
// 将温度值转换为数值
double temperature;
try {
temperature = Double.parseDouble(inputText);
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "请输入有效的温度值");
return;
}
// 判断选中的温度单位
if (fahrenheitButton.isSelected()) {
// 转换华氏度为摄氏度
double result = (temperature - 32) * 5 / 9;
outputField.setText(String.format("%.2f 摄氏度", result));
} else {
// 转换摄氏度为华氏度
double result = temperature * 9 / 5 + 32;
outputField.setText(String.format("%.2f 华氏度", result));
}
}
public static void main(String[] args) {
new TemperatureConverter();
}
}
```
该界面包含一个输入框、一个输出框、两个单选按钮和一个转换按钮。用户输入温度值后,选择华氏度或摄氏度,点击转换按钮后,程序将温度值转换为另一种单位,并将转换结果显示在输出框中。
阅读全文
相关推荐
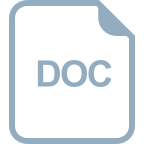
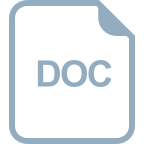
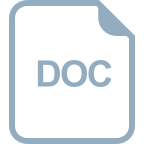
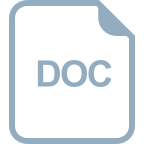
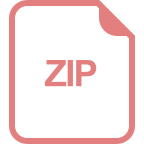
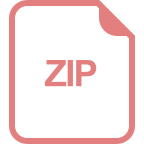
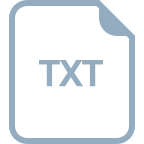
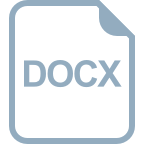
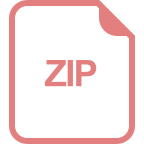
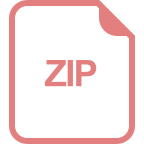
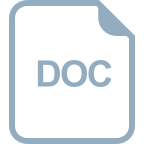
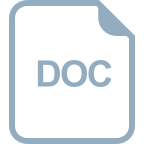
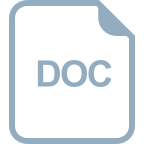
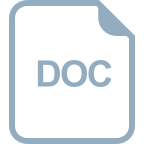

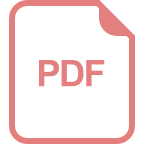
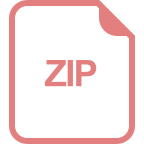