用python写一段代码,实现“已知命题公式求真值表。 输入格式: 第一行为命题公式中的命题符号个数; 第二行为命题公式。 说明: 1.公式中的命题符号不会超过4个,且从A开始依次命名; 2.公式计算顺序为先计算括号内,括号内不会出现括号,然后依次从左到右计算; 3.逻辑连接词非为!,与为^,或为+,蕴涵为>,等价为-。 输出格式: 真值表。 输入样例: 在这里给出两组输入。例如: 样例一: 2 A>B 样例二: 3 A+(B^C)”
时间: 2024-03-10 14:46:33 浏览: 72
以下是实现该功能的Python代码:
```python
def evaluate(expression, variables):
stack = []
for token in expression:
if token in variables:
stack.append(variables[token])
elif token == '!':
operand = stack.pop()
stack.append(not operand)
elif token == '^':
operand2, operand1 = stack.pop(), stack.pop()
stack.append(operand1 and operand2)
elif token == '+':
operand2, operand1 = stack.pop(), stack.pop()
stack.append(operand1 or operand2)
elif token == '>':
operand2, operand1 = stack.pop(), stack.pop()
stack.append(not operand1 or operand2)
elif token == '-':
operand2, operand1 = stack.pop(), stack.pop()
stack.append(operand1 == operand2)
return stack.pop()
def generate_truth_table(num_variables, expression):
variables = {}
for i in range(num_variables):
variables[chr(ord('A') + i)] = False
table = []
while True:
row = [variables[chr(ord('A') + i)] for i in range(num_variables)]
row.append(evaluate(expression, variables))
table.append(row)
carry = True
for i in range(num_variables):
if carry:
variables[chr(ord('A') + i)] = not variables[chr(ord('A') + i)]
carry = variables[chr(ord('A') + i)] == False
if carry:
break
return table
num_variables = int(input())
expression = input()
table = generate_truth_table(num_variables, expression)
for i in range(num_variables):
print(chr(ord('A') + i), end='\t')
print(expression)
for row in table:
for value in row[:-1]:
print(str(value), end='\t')
print(str(row[-1]))
```
你可以将上述代码保存在一个Python文件中,如“truth_table.py”,然后在命令行中运行该文件并输入命题公式的相关信息,即可输出真值表。例如,在命令行中输入以下命令:
```
python truth_table.py
2
A>B
```
程序将输出以下真值表:
```
A B A>B
False False True
False True True
True False False
True True True
```
再例如,在命令行中输入以下命令:
```
python truth_table.py
3
A+(B^C)
```
程序将输出以下真值表:
```
A B C A+(B^C)
False False False False
False False True True
False True False False
False True True True
True False False True
True False True True
True True False True
True True True True
```
阅读全文
相关推荐
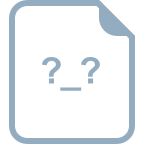
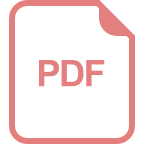

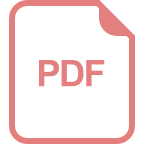
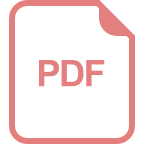
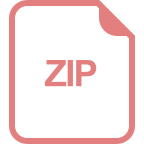
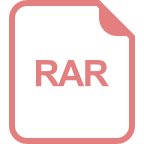
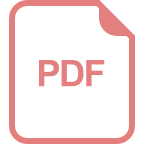
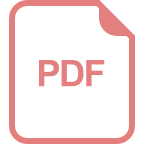
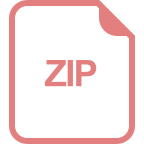
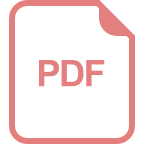
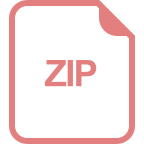
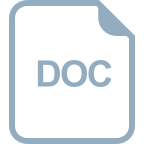
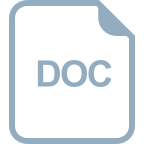
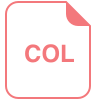


