时间片轮转调度算法的实现代码
时间: 2023-09-25 10:15:57 浏览: 88
以下是一个简单的时间片轮转调度算法的实现代码示例:
```C++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 进程控制块
struct PCB {
int pid; // 进程ID
int priority; // 进程优先级
int burst_time; // 进程需要执行的时间
int remaining_time; // 进程剩余执行时间
int arrival_time; // 进程到达时间
int finish_time; // 进程结束时间
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
// 时间片轮转调度算法
void RR_Scheduling(vector<PCB>& processes, int time_quantum) {
queue<PCB> ready_queue; // 就绪队列
int current_time = 0; // 当前时间
int n = processes.size(); // 进程数
int completed = 0; // 已完成进程数
// 将所有进程按照到达时间排序,加入就绪队列
sort(processes.begin(), processes.end(), [](const PCB& a, const PCB& b) {
return a.arrival_time < b.arrival_time;
});
int i = 0;
while (i < n) {
if (processes[i].arrival_time <= current_time) {
ready_queue.push(processes[i]);
i++;
}
else {
break;
}
}
// 时间片轮转调度
while (completed < n) {
if (ready_queue.empty()) {
current_time = processes[i].arrival_time;
while (i < n) {
if (processes[i].arrival_time <= current_time) {
ready_queue.push(processes[i]);
i++;
}
else {
break;
}
}
}
else {
PCB current_process = ready_queue.front();
ready_queue.pop();
int execute_time = min(time_quantum, current_process.remaining_time);
current_process.remaining_time -= execute_time;
current_time += execute_time;
if (current_process.remaining_time == 0) {
completed++;
current_process.finish_time = current_time;
current_process.turnaround_time = current_process.finish_time - current_process.arrival_time;
current_process.waiting_time = current_process.turnaround_time - current_process.burst_time;
}
else {
while (i < n) {
if (processes[i].arrival_time <= current_time) {
ready_queue.push(processes[i]);
i++;
}
else {
break;
}
}
ready_queue.push(current_process);
}
}
}
// 输出每个进程的信息
for (const auto& process : processes) {
cout << "Process " << process.pid << ":\n";
cout << " Arrival Time: " << process.arrival_time << endl;
cout << " Burst Time: " << process.burst_time << endl;
cout << " Finish Time: " << process.finish_time << endl;
cout << " Waiting Time: " << process.waiting_time << endl;
cout << " Turnaround Time: " << process.turnaround_time << endl;
}
}
int main() {
vector<PCB> processes = {
{1, 3, 10, 10, 0, 0, 0, 0},
{2, 1, 1, 1, 0, 0, 0, 0},
{3, 2, 3, 3, 0, 0, 0, 0},
{4, 4, 2, 2, 0, 0, 0, 0},
{5, 2, 5, 5, 0, 0, 0, 0}
};
int time_quantum = 2;
RR_Scheduling(processes, time_quantum);
return 0;
}
```
在这个示例代码中,我们定义了一个 `PCB` 结构体表示进程控制块,其中包含了进程的各种属性,如进程ID、优先级、需要执行的时间等等。
我们使用一个 `vector` 存储所有进程,按照到达时间排序后加入一个 `queue` 表示就绪队列。
在 `RR_Scheduling` 函数中,我们首先将到达时间不超过当前时间的进程加入就绪队列中。然后开始时间片轮转调度,每次从就绪队列中取出一个进程执行,执行时间为时间片长度和剩余执行时间的较小值。如果进程执行完毕,则将其从就绪队列中移除,并计算其各种信息,如结束时间、周转时间、等待时间等等。如果进程没有执行完毕,则将其放回就绪队列的队尾。当所有进程都执行完毕时,输出每个进程的各种信息。
在主函数中,我们定义了一个示例进程集合和时间片长度,并将它们传递给 `RR_Scheduling` 函数进行调度。
阅读全文
相关推荐
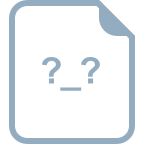
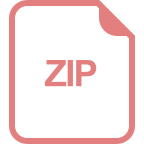
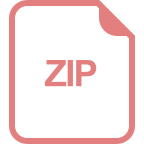
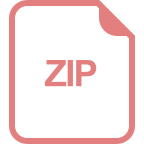
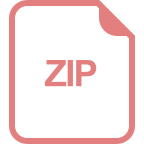
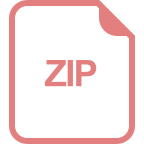
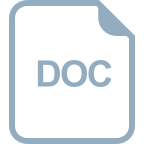
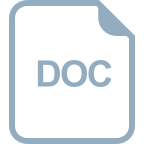
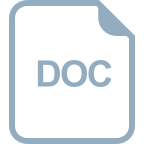
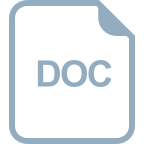
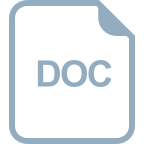
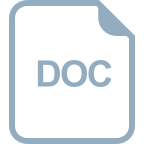
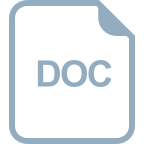
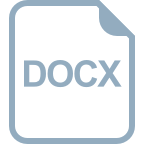
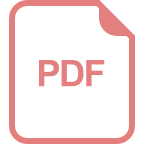