vue2 写一个温度计
时间: 2023-07-18 14:16:17 浏览: 185
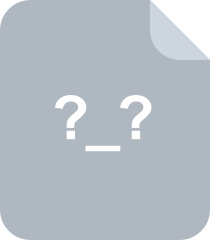
温度计编程
好的,以下是一个简单的温度计组件的代码示例,使用 Vue 2.x 来实现:
```html
<template>
<div class="thermometer">
<div class="mercury" :style="{ height: mercuryHeight }"></div>
<div class="glass"></div>
<div class="scale">
<div v-for="i in 11" :key="i" class="tick"></div>
<div class="degree" v-for="i in 10" :key="i">{{ i * 10 }}</div>
<div class="degree">℃</div>
</div>
</div>
</template>
<script>
export default {
props: {
temperature: {
type: Number,
required: true,
},
},
computed: {
mercuryHeight() {
return `${(this.temperature / 10) * 100}%`;
},
},
};
</script>
<style>
.thermometer {
position: relative;
width: 40px;
height: 200px;
margin: 20px auto;
}
.mercury {
position: absolute;
bottom: 0;
left: 0;
right: 0;
background-color: red;
transition: height 0.5s ease;
}
.glass {
position: absolute;
top: 0;
bottom: 0;
left: 10px;
right: 10px;
border: 2px solid black;
border-radius: 10px;
}
.scale {
position: absolute;
top: 0;
bottom: 0;
left: -30px;
width: 100px;
text-align: center;
font-size: 12px;
}
.tick {
position: absolute;
left: 10px;
width: 10px;
height: 2px;
background-color: black;
}
.degree {
position: absolute;
left: 20px;
width: 20px;
text-align: center;
transform: translate(-10px, 0);
}
</style>
```
上面的代码包含一个 `Thermometer` 组件,该组件接收一个 `temperature` 属性,用于表示当前温度值,以及温度计的样式。
温度计的样式使用 CSS 实现,包含一个红色的水银柱,一个黑色的玻璃管,以及一个刻度盘。在 `Thermometer` 组件中,使用计算属性 `mercuryHeight` 来动态计算水银柱的高度,并将其绑定到样式中。
在应用中使用该组件,只需要将温度值传递给 `temperature` 属性即可:
```html
<template>
<div>
<temperature :temperature="temperature"></temperature>
</div>
</template>
<script>
import Temperature from './components/Temperature.vue';
export default {
components: {
Temperature,
},
data() {
return {
temperature: 25,
};
},
};
</script>
```
阅读全文
相关推荐


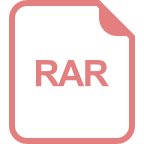
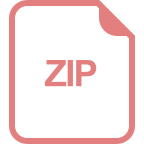
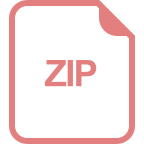
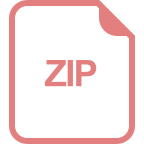
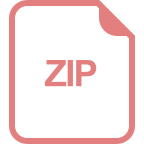
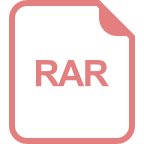
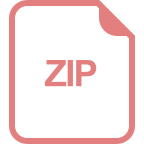
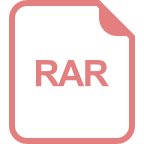
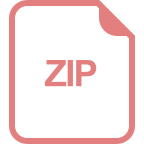
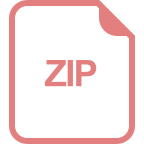
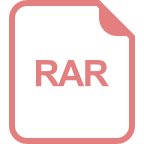
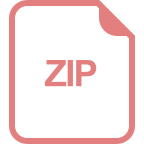
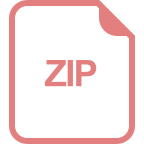
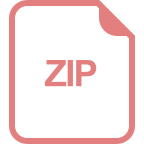