用html 和JavaScript写一个getHours(0方法、getMinutes(方法和getSecondsO方法获取当前时间的小时、分和 秒,使用定时器调用计时器函数模拟时钟特效,效果如图3.17所示。
时间: 2024-01-22 19:17:35 浏览: 26
以下是代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>时钟特效</title>
<style>
body {
background-color: #333;
}
.clock {
width: 200px;
height: 200px;
border-radius: 50%;
border: 8px solid #fff;
position: relative;
margin: 50px auto;
box-shadow: inset 0 0 10px #000, 0 0 10px #000;
}
.hour-hand, .minute-hand, .second-hand {
position: absolute;
background-color: #fff;
transform-origin: bottom center;
transition: all 0.5s ease-in-out;
}
.hour-hand {
width: 8px;
height: 60px;
top: 50px;
left: 96px;
}
.minute-hand {
width: 6px;
height: 80px;
top: 40px;
left: 97px;
}
.second-hand {
width: 4px;
height: 100px;
top: 30px;
left: 98px;
}
.mark {
position: absolute;
background-color: #fff;
border-radius: 50%;
width: 10px;
height: 10px;
left: 95px;
top: 20px;
}
.mark:nth-child(1) {
transform: rotate(30deg);
}
.mark:nth-child(2) {
transform: rotate(60deg);
}
.mark:nth-child(3) {
transform: rotate(90deg);
}
.mark:nth-child(4) {
transform: rotate(120deg);
}
.mark:nth-child(5) {
transform: rotate(150deg);
}
.mark:nth-child(6) {
transform: rotate(180deg);
}
.mark:nth-child(7) {
transform: rotate(210deg);
}
.mark:nth-child(8) {
transform: rotate(240deg);
}
.mark:nth-child(9) {
transform: rotate(270deg);
}
.mark:nth-child(10) {
transform: rotate(300deg);
}
.mark:nth-child(11) {
transform: rotate(330deg);
}
</style>
</head>
<body>
<div class="clock">
<div class="hour-hand"></div>
<div class="minute-hand"></div>
<div class="second-hand"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
<div class="mark"></div>
</div>
<script>
function clock() {
const date = new Date();
const hour = date.getHours();
const minute = date.getMinutes();
const second = date.getSeconds();
const hourHand = document.querySelector('.hour-hand');
const minuteHand = document.querySelector('.minute-hand');
const secondHand = document.querySelector('.second-hand');
hourHand.style.transform = `rotate(${hour * 30 + minute / 2}deg)`;
minuteHand.style.transform = `rotate(${minute * 6}deg)`;
secondHand.style.transform = `rotate(${second * 6}deg)`;
}
setInterval(clock, 1000);
</script>
</body>
</html>
```
代码解释:
1. 使用 HTML 和 CSS 创建一个时钟外壳,其中 `.clock` 类表示时钟本身,`.hour-hand`、`.minute-hand` 和 `.second-hand` 类表示时针、分针和秒针,`.mark` 类表示刻度。
2. 使用 JavaScript 中的 `Date` 对象获取当前时间的小时、分和秒。
3. 使用 `querySelector` 方法选择时针、分针和秒针的元素,并使用 `transform` 属性将它们旋转到正确的位置。
4. 使用 `setInterval` 方法每秒钟调用一次 `clock` 函数,以更新时钟的指针位置。
相关推荐
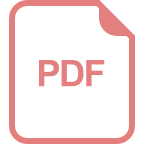
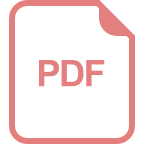
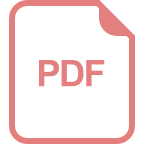














