用python开发项目,并希望使用Google test进行测试,如何配置
时间: 2023-06-26 22:05:28 浏览: 98
如果你想在Python项目中使用Google Test进行测试,可以使用Pybind11库来将C++测试代码与Python代码集成在一起。Pybind11是一个轻量级的头文件库,可以将C++函数和类转换为Python可调用的形式。
以下是一些基本步骤:
1. 首先,确保你已经安装好了Google Test和Pybind11库。
2. 在你的C++测试代码中,使用Google Test框架编写测试用例。
3. 使用Pybind11库将C++测试代码包装为Python模块。
4. 在你的Python项目中导入包装后的C++模块,并调用其中的测试函数。
以下是一个简单的示例:
假设你的C++测试代码如下所示:
```c++
#include <gtest/gtest.h>
TEST(MyTestSuite, MyTestCase) {
EXPECT_EQ(2 + 2, 4);
}
int main(int argc, char **argv) {
testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
```
然后,你可以使用Pybind11将其包装为Python模块:
```c++
#include <pybind11/pybind11.h>
#include <pybind11/stl.h>
#include <gtest/gtest.h>
namespace py = pybind11;
PYBIND11_MODULE(gtest_module, m) {
m.def("run_tests", []() {
int argc = 1;
char *argv[1] = { const_cast<char*>("gtest_module") };
testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
});
}
TEST(MyTestSuite, MyTestCase) {
EXPECT_EQ(2 + 2, 4);
}
```
在上面的代码中,我们将测试函数`run_tests`包装为Python模块,并在其中初始化Google Test并运行测试。
最后,在你的Python代码中,你可以导入模块并调用测试函数:
```python
import gtest_module
gtest_module.run_tests()
```
这将在Python中运行C++测试用例并输出结果。
相关推荐
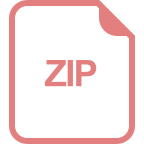
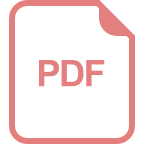
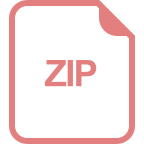
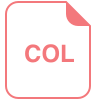
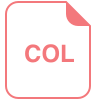
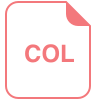
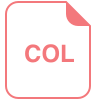
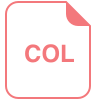









