用c++编写,实现一个布局合理美观的员工信息输入界面,要求输入姓名、工资、地址,学历、工作部门,分别用到label,lineEdit,按钮(输入,退出,打印)等控件,并将数据内容录入到数据库中,数据表单为tem.db。创建一个包含职工基本信息的数据库employee.db。字段包括整数主键id,字符型(20)name,整形 salary,字符型〈30) address等。其中输入按钮将界面中的数据内容录入到指定数据库中,退出按钮退出系统,打印按钮将数据库的数据通过qde
时间: 2024-03-12 11:44:04 浏览: 21
好的,我可以给你提供一份C++代码示例,不过需要你先安装Qt库和sqlite3库。
下面是示例代码:
```cpp
#include <QtWidgets>
#include <QtSql>
#include <sqlite3.h>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建主窗口
QWidget *mainWindow = new QWidget;
mainWindow->setWindowTitle("员工信息输入界面");
// 创建控件
QLabel *nameLabel = new QLabel("姓名:");
QLineEdit *nameLineEdit = new QLineEdit;
QLabel *salaryLabel = new QLabel("工资:");
QLineEdit *salaryLineEdit = new QLineEdit;
QLabel *addressLabel = new QLabel("地址:");
QLineEdit *addressLineEdit = new QLineEdit;
QLabel *educationLabel = new QLabel("学历:");
QLineEdit *educationLineEdit = new QLineEdit;
QLabel *departmentLabel = new QLabel("工作部门:");
QLineEdit *departmentLineEdit = new QLineEdit;
QPushButton *inputButton = new QPushButton("输入");
QPushButton *exitButton = new QPushButton("退出");
QPushButton *printButton = new QPushButton("打印");
// 创建布局
QGridLayout *layout = new QGridLayout;
layout->addWidget(nameLabel, 0, 0);
layout->addWidget(nameLineEdit, 0, 1);
layout->addWidget(salaryLabel, 1, 0);
layout->addWidget(salaryLineEdit, 1, 1);
layout->addWidget(addressLabel, 2, 0);
layout->addWidget(addressLineEdit, 2, 1);
layout->addWidget(educationLabel, 3, 0);
layout->addWidget(educationLineEdit, 3, 1);
layout->addWidget(departmentLabel, 4, 0);
layout->addWidget(departmentLineEdit, 4, 1);
layout->addWidget(inputButton, 5, 0);
layout->addWidget(exitButton, 5, 1);
layout->addWidget(printButton, 5, 2);
// 设置主窗口布局
mainWindow->setLayout(layout);
// 创建数据库连接
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("tem.db");
if (!db.open()) {
qWarning() << "无法打开数据库";
return -1;
}
// 创建数据表
QSqlQuery query;
query.exec("CREATE TABLE IF NOT EXISTS employee ("
"id INTEGER PRIMARY KEY,"
"name VARCHAR(20),"
"salary INTEGER,"
"address VARCHAR(30)"
")");
// 处理按钮点击事件
QObject::connect(inputButton, &QPushButton::clicked, [=]() {
// 获取输入的数据
QString name = nameLineEdit->text();
int salary = salaryLineEdit->text().toInt();
QString address = addressLineEdit->text();
// 插入数据到数据库
QSqlQuery query;
query.prepare("INSERT INTO employee (name, salary, address) VALUES (:name, :salary, :address)");
query.bindValue(":name", name);
query.bindValue(":salary", salary);
query.bindValue(":address", address);
query.exec();
// 清空输入框
nameLineEdit->clear();
salaryLineEdit->clear();
addressLineEdit->clear();
});
QObject::connect(exitButton, &QPushButton::clicked, [=]() {
// 退出程序
qApp->exit();
});
QObject::connect(printButton, &QPushButton::clicked, [=]() {
// 打印数据库中的数据
QSqlTableModel *model = new QSqlTableModel;
model->setTable("employee");
model->select();
QTableView *view = new QTableView;
view->setModel(model);
view->show();
});
// 显示主窗口
mainWindow->show();
// 运行程序
return app.exec();
}
```
这个示例代码中,我们使用了Qt提供的控件和布局,通过QSqlDatabase类连接sqlite数据库,并在按钮点击事件中操作数据库。你可以参考这份代码实现你的需求,不过需要注意的是,这里使用的是sqlite数据库,如果你打算使用其他数据库,可能需要修改部分代码。
相关推荐
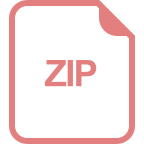
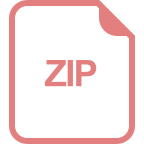
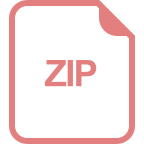














