写C程序 对输入键盘的两个字符串比较,然后输出两个字符串中第一个不相同字符的ASCII码之差
时间: 2024-12-23 22:16:08 浏览: 2
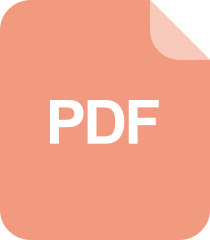
在C语言中比较两个字符串是否相等的方法
在C程序中,你可以通过以下步骤实现这个功能:
1. 首先,你需要从用户那里获取两个字符串作为输入。可以使用`fgets()`函数读取键盘输入,并存储到两个字符数组中。
```c
#include <stdio.h>
#include <string.h>
char str1[50], str2[50];
int main() {
printf("请输入第一个字符串: ");
fgets(str1, sizeof(str1), stdin);
str1[strcspn(str1, "\n")] = '\0'; // 去除行尾的换行符
printf("请输入第二个字符串: ");
fgets(str2, sizeof(str2), stdin);
str2[strcspn(str2, "\n")] = '\0'; // 去除行尾的换行符
}
```
2. 接下来,遍历两个字符串,查找第一个不相同的字符。你可以使用`strcmp()`函数比较字符,如果返回值不是0,则说明找到了不相同的字符。
```c
int first_diff_index = -1; // 初始化差异字符索引为-1
for (int i = 0; i < strlen(str1); i++) {
if (str1[i] != str2[i]) {
first_diff_index = i;
break;
}
}
if (first_diff_index == -1) { // 如果整个字符串都相同,检查长度
if (strlen(str1) > strlen(str2)) {
first_diff_index = strlen(str2);
} else {
first_diff_index = strlen(str1);
}
}
```
3. 最后,计算并输出ASCII码之差。如果是两个不同的字符,`str1[first_diff_index]`和`str2[first_diff_index]`就是不相同的字符,它们的ASCII码之差可以直接相减。
```c
if (first_diff_index != -1) {
int ascii_diff = (int)str1[first_diff_index] - (int)str2[first_diff_index];
printf("第一个不相同的字符 '%c' 和 '%c' 的 ASCII 码之差是 %d\n", str1[first_diff_index], str2[first_diff_index], ascii_diff);
} else {
printf("两个字符串完全相同。\n");
}
```
阅读全文
相关推荐
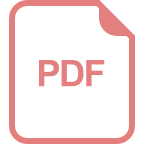
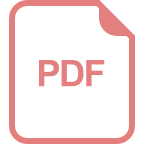















