byte[] sourceArray = new byte[10]; // 源数组 byte[] destinationArray = new byte[5]; // 目标数组 // 使用 Array.Copy 方法进行拷贝 Array.Copy(sourceArray[5], destinationArray, 5);我要指定从sourceArray这个数组的第5个元素开始,要怎么实现?
时间: 2024-04-16 12:27:11 浏览: 50
如果你想从 `sourceArray` 数组的第5个元素开始拷贝5个元素到 `destinationArray` 数组中,你可以使用 `Array.Copy` 方法的重载版本。这个重载版本接受两个起始索引作为参数,指定源数组和目标数组的起始位置。
下面是修改后的示例代码:
```csharp
byte[] sourceArray = new byte[10]; // 源数组
byte[] destinationArray = new byte[5]; // 目标数组
// 使用 Array.Copy 方法进行拷贝
Array.Copy(sourceArray, 5, destinationArray, 0, 5);
```
在这个示例中,我们使用 `Array.Copy` 方法的重载版本,指定了源数组 `sourceArray` 的起始索引为5,目标数组 `destinationArray` 的起始索引为0,并且拷贝了5个元素。
注意,这里的索引是从0开始的,所以第5个元素的索引是4(因为数组索引是从0开始计数)。
希望这次能对你有所帮助。如果还有其他问题,请随时提问。
相关问题
使用Scala 优化以下代码 def copyDirectory(source: File, destination: File): Unit = { if (source.isDirectory) { if (!destination.exists()) { destination.mkdir() } val files = source.listFiles() for (f <- files) { val newFile = new File(destination.getAbsolutePath + File.separator + f.getName) copyDirectory(f, newFile) } } else { val in = new FileInputStream(source) val out = new FileOutputStream(destination) val buffer = new Array[Byte](1024) var length = in.read(buffer) while (length > 0) { out.write(buffer, 0, length) length = in.read(buffer) } in.close() out.close() } }
以下是对 `copyDirectory` 方法进行优化的示例代码:
```scala
import java.io._
object FileCopy {
def main(args: Array[String]): Unit = {
val source = new File("path/to/source/directory")
val destination = new File("path/to/destination/directory")
copyDirectory(source, destination)
}
def copyDirectory(source: File, destination: File): Unit = {
if (!source.exists()) {
return
}
if (source.isDirectory) {
if (!destination.exists()) {
destination.mkdir()
}
val files = Option(source.listFiles()).getOrElse(Array.empty[File])
for (f <- files) {
val newFile = new File(destination, f.getName)
copyDirectory(f, newFile)
}
} else {
val in = new FileInputStream(source)
val out = new FileOutputStream(destination)
try {
val buffer = new Array[Byte](4096)
var bytesRead = in.read(buffer)
while (bytesRead != -1) {
out.write(buffer, 0, bytesRead)
bytesRead = in.read(buffer)
}
} finally {
in.close()
out.close()
}
}
}
}
```
在上述代码中,我们首先添加了一个条件判断,如果源文件或目录不存在,则直接返回。接着,我们使用 `Option` 对 `source.listFiles()` 方法的返回值进行了安全处理,如果为空则返回一个空数组。这样可以避免在遍历文件时出现空指针异常。另外,我们使用了 `File` 的构造函数来创建新文件对象,而不是使用字符串拼接的方式。这样可以提高代码的可读性。
在文件复制的部分,我们使用了 try-finally 语句块来确保输入和输出流都能被正确地关闭。此外,我们将缓冲区的大小增加到了 4096 字节,这样可以提高文件复制的效率。
g_byte_array_new
g_byte_array_new是GLib库中的一个函数,用于创建一个新的GByteArray字节数组对象。\[1\]该函数没有参数,返回一个指向新创建的字节数组对象的指针。这个字节数组对象的初始长度为0,没有分配任何内存空间。可以使用其他函数来填充、修改和释放这个字节数组对象。\[2\]
#### 引用[.reference_title]
- *1* *3* [4_05_GLib库入门与实践_字节数组](https://blog.csdn.net/field1003/article/details/123435850)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Glib学习(8) 动态字节数组 Byte Arrays](https://blog.csdn.net/andylauren/article/details/51429781)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
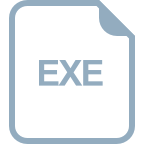
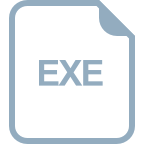













