javascript在随机背景色的基础上加上一串文字,文字的颜色是与随机生成的背景色是相反的
时间: 2024-09-26 22:04:24 浏览: 29
在JavaScript中,你可以通过以下步骤来创建一个动态网页,其中背景颜色是随机生成的,并且文字颜色与之形成对比:
1. 首先,你需要获取一个随机颜色值。可以使用`Math.random()`函数配合数组索引来生成RGB颜色。例如:
```javascript
function getRandomColor() {
const letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
```
2. 然后,根据这个随机颜色,计算其互补色(通常是对比色)。这里可以用到CSS的一些颜色理论,比如取反、转换十六进制等。不过JavaScript本身并不直接支持这种操作,所以需要额外处理:
```javascript
function getContrastColor(hex) {
// ...编写一段代码(可能使用库如tinycolor2)来计算互补色
// 对于简单的应用,你可以使用类似下面的简单方法,仅作演示:
hex = hex.replace('#', '');
const r = parseInt(hex.substr(0, 2), 16);
const g = parseInt(hex.substr(2, 2), 16);
const b = parseInt(hex.substr(4, 2), 16);
return `#${(0xFF - r).toString(16).padStart(2, '0')}${(0xFF - g).toString(16).padStart(2, '0')}${(0xFF - b).toString(16).padStart(2, '0')}`;
}
const backgroundColor = getRandomColor();
const textColor = getContrastColor(backgroundColor);
```
3. 最后,在HTML中应用这两个颜色:
```html
<body style="background-color: ${backgroundColor}; color: ${textColor}">
<h1>Hello, World!</h1>
</body>
```
阅读全文
相关推荐
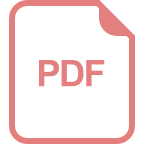
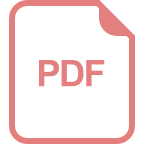
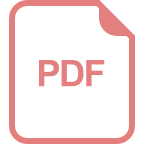
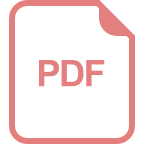


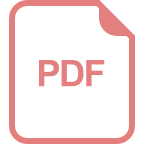
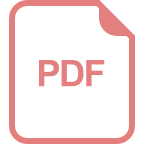
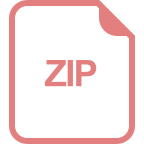
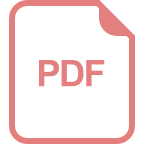
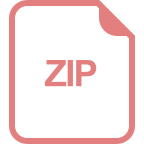
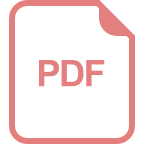
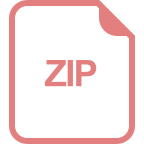
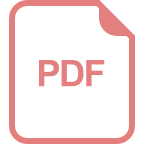
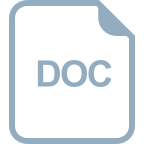
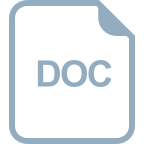

