采用正则表达式获取html_doc.html文件中的所有<a>标签下的 class、href、id属性并保存成csv文件。
时间: 2024-10-15 20:24:04 浏览: 39
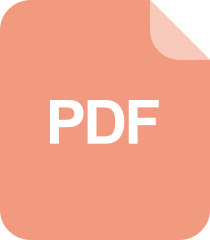
Java基于正则表达式获取指定HTML标签指定属性值的方法
可以使用Python的BeautifulSoup库解析HTML文件,并结合re模块(正则表达式)来提取<a>标签下的class、href和id属性。首先确保已安装这两个库(`beautifulsoup4`和`lxml`),然后按照以下步骤操作:
1. 导入必要的库:
```python
import csv
from bs4 import BeautifulSoup
import re
```
2. 读取HTML文件:
```python
with open('html_doc.html', 'r') as file:
html_content = file.read()
```
3. 使用BeautifulSoup解析HTML内容:
```python
soup = BeautifulSoup(html_content, 'lxml')
```
4. 定义正则表达式模式来匹配class、href和id属性:
```python
attr_patterns = {
'class': r'class="(.*?)"',
'href': r'href="(.*?)"',
'id': r'id="(.*?)"'
}
```
5. 遍历所有的<a>标签并提取所需属性:
```python
results = []
for a_tag in soup.find_all('a'):
for attr, pattern in attr_patterns.items():
match = re.search(pattern, str(a_tag))
if match:
results.append((attr, match.group(1)))
```
6. 将结果保存到CSV文件:
```python
with open('output.csv', 'w', newline='') as csvfile:
fieldnames = ['Attribute', 'Value']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(results)
```
阅读全文
相关推荐
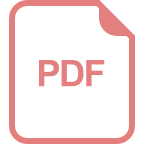















