C# winform窗体 启动winform,根据winform的大小调整电脑屏幕的分辨率 关闭winform恢复电脑屏幕原来的分辨率
时间: 2024-10-30 08:07:13 浏览: 30
在C# WinForms中,您可以使用以下步骤来实现根据WinForm大小调整电脑屏幕分辨率,并在关闭WinForm后恢复原始分辨率的功能:
1. 在WinForm窗体中添加一个窗体级别的事件处理程序,例如窗体加载事件(FormLoad)或窗体关闭事件(FormClosing)。
2. 在窗体加载事件中,获取当前屏幕分辨率并存储在变量中。您可以使用System.Windows.Forms.Screen类的GetBounds方法获取屏幕分辨率。
3. 在WinForm窗体的大小调整时,根据新的大小调整屏幕分辨率。您可以使用System.Windows.Forms.Control类的SetBounds方法来调整WinForm的大小,并使用SetDesktopResolution方法来设置新的屏幕分辨率。请注意,您需要获取当前桌面环境的桌面分辨率来确保设置的有效性。
```csharp
public partial class Form1 : Form
{
private Screen screen;
private int screenWidth;
private int screenHeight;
public Form1()
{
InitializeComponent();
screen = Screen.FromHandle(this.Handle);
screenWidth = screen.Bounds.Width;
screenHeight = screen.Bounds.Height;
}
private void Form1_Load(object sender, EventArgs e)
{
// 获取当前屏幕分辨率并存储在变量中
this.Size = new Size(screenWidth, screenHeight);
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
// 在关闭WinForm之前恢复原始分辨率
this.SetDesktopResolution(screenWidth, screenHeight);
}
private void Form1_Resize(object sender, EventArgs e)
{
// 根据WinForm大小调整屏幕分辨率
this.SetBounds(this.Location, this.Size);
}
}
```
4. 在`SetDesktopResolution`方法中,使用以下代码将屏幕分辨率设置为新的值:
```csharp
public void SetDesktopResolution(int width, int height)
{
var desktopEnvironment = SystemInformation.DesktopWidth; // 获取当前桌面环境的桌面分辨率
int desktopAspectRatio = width * height; // 获取屏幕分辨率的宽高比
var currentScreenAspectRatio = SystemInfo.PrimaryMonitorArea.Width * SystemInfo.PrimaryMonitorArea.Height; // 获取当前屏幕的宽高比
if (currentScreenAspectRatio == desktopAspectRatio) // 如果当前屏幕的宽高比与桌面环境一致,则无需调整分辨率
return;
else if (currentScreenAspectRatio > desktopAspectRatio) // 如果当前屏幕的宽高比大于桌面环境,则需要缩小屏幕分辨率以适应当前屏幕大小
width = SystemInfo.PrimaryMonitorArea.Width; // 缩小宽度为当前屏幕宽度
else // 如果当前屏幕的宽高比小于桌面环境,则需要放大屏幕分辨率以适应当前屏幕大小
height = SystemInfo.PrimaryMonitorArea.Height; // 放大高度为当前屏幕高度
System.Windows.Forms.Application.Screen.SetResolution(width, height); // 设置新的屏幕分辨率
}
```
这样,当您启动WinForm窗体时,它会根据大小调整电脑屏幕的分辨率。当您关闭WinForm窗体时,它会恢复原始分辨率。请注意,这种方法仅适用于单个WinForm窗体,如果您有多个窗体需要调整分辨率,您需要为每个窗体添加相应的处理程序。
阅读全文
相关推荐





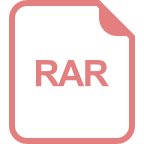
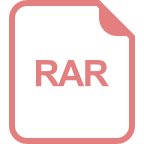
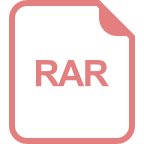







