编程创建2个子进程,子进程1运行指定路径下的可执行文件(如:/home/user/birthday),子进程2暂停10s之后退出,父进程先用阻塞方式等待子进程1的结束,然后用非阻塞方式等待子进程2的结束,待收集到二个子进程结束的信息,父进程就返回。
时间: 2023-05-26 18:06:16 浏览: 92
下面是一个示例程序,使用了fork、exec等系统调用来创建子进程并执行可执行文件,以及waitpid等系统调用来等待子进程结束。
```C
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid1, pid2;
int status;
char *args[] = {"/home/user/birthday", NULL}; // 子进程1要执行的可执行文件路径
pid1 = fork();
if (pid1 < 0) { // 失败
perror("fork");
exit(1);
} else if (pid1 == 0) { // 子进程1
if (execv(args[0], args) < 0) { // 执行可执行文件
perror("execv");
exit(1);
}
}
pid2 = fork();
if (pid2 < 0) { // 失败
perror("fork");
exit(1);
} else if (pid2 == 0) { // 子进程2
sleep(10); // 暂停10s
exit(0);
}
// 父进程
// 等待子进程1结束
if (waitpid(pid1, &status, 0) < 0) {
perror("waitpid");
exit(1);
}
// 非阻塞方式等待子进程2结束
while (waitpid(pid2, &status, WNOHANG) == 0) {
sleep(1); // 暂停1s
}
return 0;
}
```
相关推荐
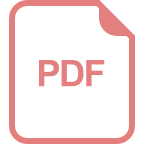
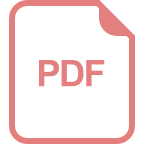
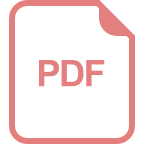














