使用html+css+js写一个抽奖转盘
时间: 2023-09-02 14:06:20 浏览: 130
好的,以下是一个使用HTML、CSS和JS实现的抽奖转盘:
HTML代码:
```html
<div class="container">
<div class="wheel">
<div class="section"><span>1</span></div>
<div class="section"><span>2</span></div>
<div class="section"><span>3</span></div>
<div class="section"><span>4</span></div>
<div class="section"><span>5</span></div>
<div class="section"><span>6</span></div>
</div>
<button class="btn" onclick="start()">开始抽奖</button>
</div>
```
CSS代码:
```css
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f2f2f2;
}
.wheel {
position: relative;
width: 300px;
height: 300px;
border-radius: 50%;
background-color: #ff9966;
overflow: hidden;
transform: rotate(0deg);
transition: transform 6s ease-in-out;
}
.section {
position: absolute;
width: 100%;
height: 100%;
text-align: center;
font-size: 30px;
font-weight: bold;
color: #fff;
line-height: 300px;
transition: transform 1s ease-in-out;
}
.section:nth-child(1) {
transform: rotate(0deg);
background-color: #ffcc99;
}
.section:nth-child(2) {
transform: rotate(60deg);
background-color: #ff9933;
}
.section:nth-child(3) {
transform: rotate(120deg);
background-color: #ffcc33;
}
.section:nth-child(4) {
transform: rotate(180deg);
background-color: #ff6633;
}
.section:nth-child(5) {
transform: rotate(240deg);
background-color: #ff9933;
}
.section:nth-child(6) {
transform: rotate(300deg);
background-color: #ffcc99;
}
.btn {
margin-top: 30px;
padding: 10px 20px;
font-size: 20px;
font-weight: bold;
color: #fff;
background-color: #ff9933;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease-in-out;
}
.btn:hover {
background-color: #ffcc33;
}
```
JS代码:
```javascript
var deg = 0;
var speed = 0;
var prize = '';
function start() {
// 生成一个随机速度
speed = Math.floor(Math.random() * 20) + 10;
// 生成一个随机奖品
prize = Math.floor(Math.random() * 6) + 1;
// 计算需要旋转的角度
deg = 360 / 6 * (prize - 1) + 360 / 6 / 2 - Math.random() * 360 / 6;
// 开始旋转转盘
var wheel = document.querySelector('.wheel');
wheel.style.transform = 'rotate(' + deg + 'deg)';
wheel.style.transition = 'transform 6s ease-in-out';
// 6秒后停止旋转
setTimeout(function() {
stop();
}, 6000);
}
function stop() {
// 停止旋转
var wheel = document.querySelector('.wheel');
wheel.style.transform = 'rotate(' + deg + 'deg)';
wheel.style.transition = 'transform 0s';
// 显示中奖结果
alert('恭喜您获得:' + document.querySelector('.section:nth-child(' + prize + ') span').innerHTML);
}
```
这个抽奖转盘使用了CSS3的旋转效果来实现转盘的转动,使用JS生成随机速度和奖品,并计算需要旋转的角度。在点击开始抽奖按钮后,将转盘旋转到指定角度,并在6秒后停止旋转,并弹出中奖结果提示框。
相关推荐
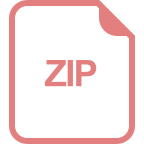
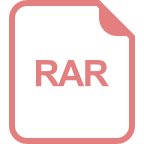
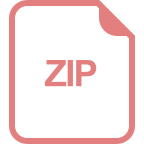













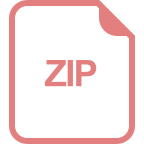
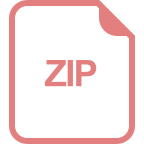