使用xamarin.forms实现蓝牙搜索、连接
时间: 2023-12-26 19:03:32 浏览: 161
要实现蓝牙搜索和连接,可以使用Xamarin.Essentials库。以下是一些基本步骤:
1. 添加Xamarin.Essentials库到Xamarin.Forms项目中。
2. 在Xamarin.Forms界面中添加一个按钮,用于开始蓝牙搜索。
3. 在按钮的点击事件中,调用以下代码启动蓝牙搜索:
```
var results = await BluetoothLE.ScanAsync();
foreach (var result in results)
{
// 处理搜索结果
}
```
4. 处理搜索结果,找到需要连接的设备,然后调用以下代码连接设备:
```
var device = await BluetoothLE.ConnectAsync(result.Device);
```
5. 连接成功后,可以使用以下代码读取和写入数据:
```
var service = await device.GetServiceAsync(serviceId);
var characteristic = await service.GetCharacteristicAsync(characteristicId);
var value = await characteristic.ReadAsync();
await characteristic.WriteAsync(data);
```
以上步骤仅为基本示例,实际使用中需要根据具体需求进行修改和完善。
相关问题
用xamarin.forms 连接蓝牙,发送zpl指令打印图片
首先,您需要添加Xamarin.Essentials和Xamarin.Forms.PrintSupport NuGet包到您的项目中。然后,您可以使用以下代码连接到蓝牙打印机:
```csharp
// 获取可用的蓝牙设备列表
var devices = await BluetoothService.GetPairedDevicesAsync();
// 过滤出您的蓝牙打印机
var printer = devices.FirstOrDefault(d => d.Name == "YourPrinterName");
// 如果找到了打印机,则连接
if (printer != null)
{
var service = new BluetoothService(printer);
await service.ConnectAsync();
// 发送ZPL指令
var zpl = "^XA^FO20,20^XGR:IMAGE.GRF,1,1^FS^XZ";
var data = Encoding.UTF8.GetBytes(zpl);
await service.SendAsync(data);
// 断开连接
service.Disconnect();
}
```
在上面的代码中,我们使用了Xamarin.Essentials中的`BluetoothService`类来连接到蓝牙打印机。然后,我们使用ZPL指令来打印图片,将其转换为字节数组并通过`SendAsync`方法发送。最后,我们使用`Disconnect`方法断开连接。
请注意,您需要将`IMAGE.GRF`替换为您实际的图片文件名,并将其上传到打印机中。此外,您可能需要调整ZPL指令以适应您的打印机和图片大小。
xamarin.forms 蓝牙打印
Xamarin.Forms可以使用BluetoothPrinter插件实现蓝牙打印功能。
1. 在Xamarin.Forms项目中安装BluetoothPrinter插件:
在NuGet包管理器中搜索并安装BluetoothPrinter插件。
2. 创建蓝牙打印机连接:
```csharp
using BluetoothPrinter;
using System.Threading.Tasks;
public async Task<bool> ConnectBluetoothPrinter(string macAddress)
{
var printer = new BluetoothPrinterManager(macAddress);
var connectResult = await printer.ConnectAsync();
if (connectResult)
{
// 连接成功
return true;
}
else
{
// 连接失败
return false;
}
}
```
3. 打印文本:
```csharp
public async Task PrintText(string macAddress, string text)
{
var printer = new BluetoothPrinterManager(macAddress);
var connectResult = await printer.ConnectAsync();
if (connectResult)
{
// 连接成功,打印文本
await printer.PrintTextAsync(text);
printer.Disconnect();
}
}
```
4. 打印图片:
```csharp
public async Task PrintImage(string macAddress, string imagePath)
{
var printer = new BluetoothPrinterManager(macAddress);
var connectResult = await printer.ConnectAsync();
if (connectResult)
{
// 连接成功,打印图片
await printer.PrintImageAsync(imagePath);
printer.Disconnect();
}
}
```
注意:在Android项目中需要添加以下权限:
```xml
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
```
阅读全文
相关推荐
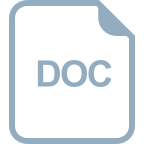
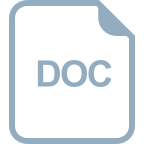
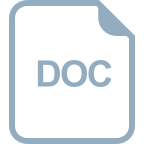
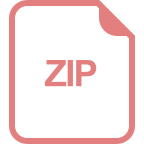
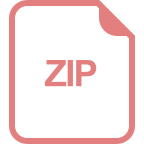
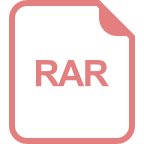
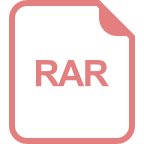
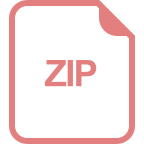
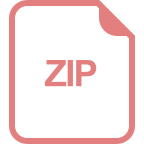
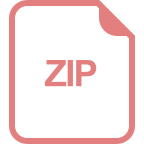
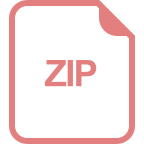
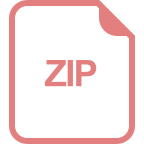
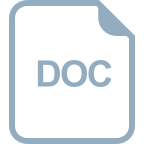
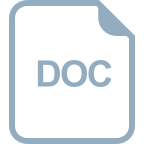
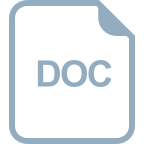
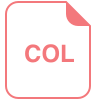
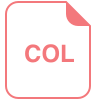