c语言红黑树基本操作,请用代码展示
时间: 2023-05-21 09:03:13 浏览: 65
以下是C语言红黑树的基本操作代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef enum { RED, BLACK } nodeColor;
typedef struct _node {
int key;
nodeColor color;
struct _node *left;
struct _node *right;
struct _node *parent;
} node;
node *root = NULL;
node *createNode(int key) {
node *newNode = (node *)malloc(sizeof(node));
newNode->key = key;
newNode->color = RED;
newNode->left = NULL;
newNode->right = NULL;
newNode->parent = NULL;
return newNode;
}
void rotateLeft(node *x) {
node *y = x->right;
x->right = y->left;
if (y->left != NULL) {
y->left->parent = x;
}
y->parent = x->parent;
if (x->parent == NULL) {
root = y;
} else if (x == x->parent->left) {
x->parent->left = y;
} else {
x->parent->right = y;
}
y->left = x;
x->parent = y;
}
void rotateRight(node *x) {
node *y = x->left;
x->left = y->right;
if (y->right != NULL) {
y->right->parent = x;
}
y->parent = x->parent;
if (x->parent == NULL) {
root = y;
} else if (x == x->parent->right) {
x->parent->right = y;
} else {
x->parent->left = y;
}
y->right = x;
x->parent = y;
}
void fixInsert(node *z) {
while (z->parent != NULL && z->parent->color == RED) {
if (z->parent == z->parent->parent->left) {
node *y = z->parent->parent->right;
if (y != NULL && y->color == RED) {
z->parent->color = BLACK;
y->color = BLACK;
z->parent->parent->color = RED;
z = z->parent->parent;
} else {
if (z == z->parent->right) {
z = z->parent;
rotateLeft(z);
}
z->parent->color = BLACK;
z->parent->parent->color = RED;
rotateRight(z->parent->parent);
}
} else {
node *y = z->parent->parent->left;
if (y != NULL && y->color == RED) {
z->parent->color = BLACK;
y->color = BLACK;
z->parent->parent->color = RED;
z = z->parent->parent;
} else {
if (z == z->parent->left) {
z = z->parent;
rotateRight(z);
}
z->parent->color = BLACK;
z->parent->parent->color = RED;
rotateLeft(z->parent->parent);
}
}
}
root->color = BLACK;
}
void insert(int key) {
node *z = createNode(key);
node *y = NULL;
node *x = root;
while (x != NULL) {
y = x;
if (z->key < x->key) {
x = x->left;
} else {
x = x->right;
}
}
z->parent = y;
if (y == NULL) {
root = z;
} else if (z->key < y->key) {
y->left = z;
} else {
y->right = z;
}
fixInsert(z);
}
void inorderTraversal(node *x) {
if (x != NULL) {
inorderTraversal(x->left);
printf("%d ", x->key);
inorderTraversal(x->right);
}
}
int main() {
insert(10);
insert(20);
insert(30);
insert(15);
insert(18);
insert(25);
insert(40);
printf("Inorder Traversal: ");
inorderTraversal(root);
printf("\n");
return 0;
}
```
以上代码实现了红黑树的插入操作,并且在插入节点后进行了修复操作,以保证红黑树的性质。
相关推荐
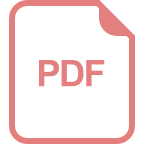
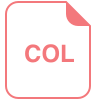
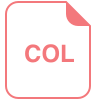
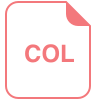
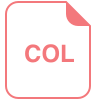











