uniapp怎么获取遍历的数据的全部dom元素
时间: 2023-06-12 18:05:30 浏览: 86
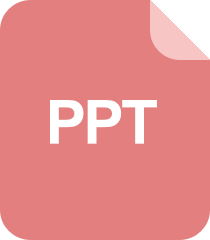
jQuery ligerUI API chm
在uniapp中,可以使用wx.createSelectorQuery()方法获取遍历数据的全部dom元素。具体步骤如下:
1. 在需要遍历数据的页面中,使用wx.createSelectorQuery()创建一个查询对象。
2. 调用查询对象的selectAll()方法,传入需要遍历的选择器,例如".list-item"。
3. 调用查询对象的boundingClientRect()方法,获取每个元素的位置和大小信息。
4. 调用查询对象的exec()方法,执行查询操作,并在回调函数中获取查询结果。
代码示例:
```
// 获取所有class为list-item的元素
wx.createSelectorQuery().selectAll('.list-item').boundingClientRect((rects) => {
// rects是一个数组,包含每个元素的位置和大小信息
rects.forEach((rect) => {
console.log(rect)
})
}).exec()
```
阅读全文
相关推荐
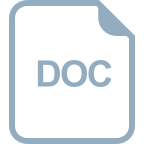
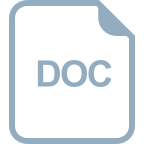
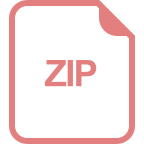
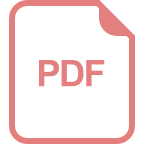



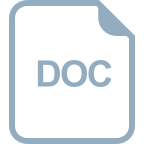
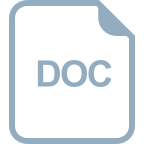
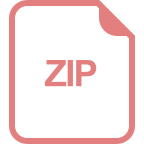
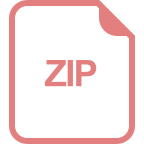
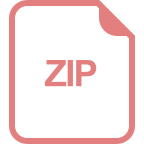
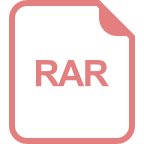
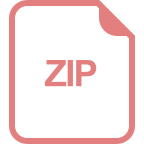