如何将assertion集成到uvm环境中的例子
时间: 2024-02-17 08:02:54 浏览: 18
以下是一个将assertion集成到UVM环境中的例子:
1. 创建一个UVM Assertion Component(UVM AC):
```verilog
class my_assertion_component extends uvm_component;
`uvm_component_utils(my_assertion_component)
function new(string name, uvm_component parent);
super.new(name, parent);
endfunction
task run_phase(uvm_phase phase);
// 在这里添加assertion
endtask
endclass
```
2. 在UVM testbench中实例化UVM AC组件,并将其与其他组件连接起来:
```verilog
class my_testbench extends uvm_test;
`uvm_component_utils(my_testbench)
my_assertion_component my_assertion_comp;
function new(string name, uvm_component parent);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
// 实例化UVM AC组件
my_assertion_comp = my_assertion_component::type_id::create("my_assertion_comp", this);
// 将UVM AC组件与其他组件连接起来
my_assertion_comp.my_dut = my_dut;
my_assertion_comp.my_monitor = my_monitor;
endfunction
task run_phase(uvm_phase phase);
// 启动UVM AC组件
my_assertion_comp.start();
phase.raise_objection(this);
phase.drop_objection(this);
endtask
endclass
```
3. 在UVM AC中定义assertion,并使用UVM提供的Assertion API将其添加到assertion队列中:
```verilog
class my_assertion_component extends uvm_component;
`uvm_component_utils(my_assertion_component)
function new(string name, uvm_component parent);
super.new(name, parent);
endfunction
task run_phase(uvm_phase phase);
// 定义assertion
uvm_assert_info("my_assertion", my_dut.data == my_monitor.data, "data mismatch");
// 将assertion添加到assertion队列中
uvm_check_output();
endtask
endclass
```
4. 通过UVM testbench控制assertion的启动和停止,并收集assertion的结果:
```verilog
class my_testbench extends uvm_test;
`uvm_component_utils(my_testbench)
my_assertion_component my_assertion_comp;
function new(string name, uvm_component parent);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
// 实例化UVM AC组件
my_assertion_comp = my_assertion_component::type_id::create("my_assertion_comp", this);
// 将UVM AC组件与其他组件连接起来
my_assertion_comp.my_dut = my_dut;
my_assertion_comp.my_monitor = my_monitor;
endfunction
task run_phase(uvm_phase phase);
// 启动UVM AC组件
my_assertion_comp.start();
phase.raise_objection(this);
// 等待assertion完成
uvm_wait_for_nba_region();
// 停止UVM AC组件
my_assertion_comp.stop();
// 收集assertion的结果
uvm_report_info(get_type_name(), $sformatf("Assertion summary: %0d pass, %0d fail", uvm_get_assertion_count(UVM_PASS), uvm_get_assertion_count(UVM_FAIL)), UVM_NONE);
phase.drop_objection(this);
endtask
endclass
```
5. 在仿真结束后,通过UVM report机制生成assertion的报告:
```verilog
class my_testbench extends uvm_test;
`uvm_component_utils(my_testbench)
my_assertion_component my_assertion_comp;
function new(string name, uvm_component parent);
super.new(name, parent);
endfunction
function void build_phase(uvm_phase phase);
super.build_phase(phase);
// 实例化UVM AC组件
my_assertion_comp = my_assertion_component::type_id::create("my_assertion_comp", this);
// 将UVM AC组件与其他组件连接起来
my_assertion_comp.my_dut = my_dut;
my_assertion_comp.my_monitor = my_monitor;
endfunction
task run_phase(uvm_phase phase);
// 启动UVM AC组件
my_assertion_comp.start();
phase.raise_objection(this);
// 等待assertion完成
uvm_wait_for_nba_region();
// 停止UVM AC组件
my_assertion_comp.stop();
// 收集assertion的结果
uvm_report_info(get_type_name(), $sformatf("Assertion summary: %0d pass, %0d fail", uvm_get_assertion_count(UVM_PASS), uvm_get_assertion_count(UVM_FAIL)), UVM_NONE);
// 生成assertion报告
uvm_report_summarize();
phase.drop_objection(this);
endtask
endclass
```
上述例子中,使用了UVM提供的Assertion API实现了一个简单的数据比较assertion,并通过UVM report机制生成了assertion的报告。实际应用中,还需要根据具体的需求定义更复杂的assertion,并且也需要考虑到assertion的性能问题。
相关推荐
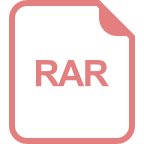
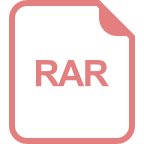














