showALLProduct.jsp里面进去展示所有商品信息,表格有四列,最前面为cherkbox复选框,product数据库表有pid,pname,categpry表有cid和cname,第二列为商品id,即pid,第三列为商品名称,即pname,第四列为类别名称,即cname,最后一列为操作,即删除按钮,有productDao类,ProductService类,BaseServlet类代码怎么写
时间: 2024-03-14 15:44:36 浏览: 97
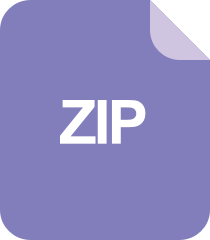
使用JSP+Servlet实现商品管理功能,后台为数据库,功能包括商品信息浏览、商品信息详情、商品信息删除及修改。

以下是代码示例:
ProductDao类:
```java
public class ProductDao {
// 根据商品ID删除商品
public boolean deleteProductById(int pid) {
// TODO: 实现删除商品的代码
return false;
}
// 查询所有商品信息
public List<Product> findAllProducts() {
// TODO: 实现查询所有商品信息的代码
return null;
}
}
```
ProductService类:
```java
public class ProductService {
private ProductDao productDao = new ProductDao();
// 删除商品
public boolean deleteProductById(int pid) {
return productDao.deleteProductById(pid);
}
// 查询所有商品信息
public List<Product> findAllProducts() {
return productDao.findAllProducts();
}
}
```
BaseServlet类:
```java
public abstract class BaseServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 设置请求编码
request.setCharacterEncoding("UTF-8");
// 设置响应编码
response.setContentType("text/html;charset=UTF-8");
// 获取操作类型
String action = request.getParameter("action");
// 根据操作类型调用相应的方法
if ("deleteProduct".equals(action)) {
deleteProduct(request, response);
} else if ("showAllProducts".equals(action)) {
showAllProducts(request, response);
}
}
// 删除商品
protected void deleteProduct(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int pid = Integer.parseInt(request.getParameter("pid"));
ProductService productService = new ProductService();
boolean result = productService.deleteProductById(pid);
if (result) {
// 删除成功,跳转到展示所有商品信息的页面
response.sendRedirect(request.getContextPath() + "/showALLProduct.jsp");
} else {
// 删除失败,返回错误信息
response.getWriter().write("删除商品失败");
}
}
// 展示所有商品信息
protected void showAllProducts(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ProductService productService = new ProductService();
List<Product> productList = productService.findAllProducts();
// 把商品信息存放到request域中,转发到展示所有商品信息的页面
request.setAttribute("productList", productList);
request.getRequestDispatcher("/showALLProduct.jsp").forward(request, response);
}
}
```
showALLProduct.jsp页面:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>展示所有商品信息</title>
</head>
<body>
<table border="1">
<thead>
<tr>
<th>选择</th>
<th>商品ID</th>
<th>商品名称</th>
<th>类别名称</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${productList}" var="product">
<tr>
<td><input type="checkbox" name="pid" value="${product.pid}"></td>
<td>${product.pid}</td>
<td>${product.pname}</td>
<td>${product.category.cname}</td>
<td><a href="${pageContext.request.contextPath}/ProductServlet?action=deleteProduct&pid=${product.pid}">删除</a></td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
以上代码示例中,ProductDao类和ProductService类分别用于处理数据库操作和业务逻辑,BaseServlet类是一个基础Servlet,用于接收请求,根据请求参数调用相应的方法处理请求,并返回响应。在showALLProduct.jsp页面中,使用了JSTL的forEach标签遍历商品信息,并用HTML表格展示。
阅读全文
相关推荐
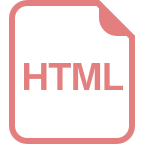






