android系统中如何实现vendor lib去调用system lib
时间: 2024-04-29 12:21:36 浏览: 11
在 Android 系统中,Vendor lib 是指供应商提供的专用库,而 System lib 是指系统默认提供的公共库。在 Vendor lib 中调用 System lib 可以通过以下步骤实现:
1. 在 Vendor lib 中定义对 System lib 的依赖。
2. 使用 dlopen() 函数加载 System lib。
3. 使用 dlsym() 函数获取 System lib 中的函数指针。
4. 使用获取到的函数指针调用 System lib 中的函数。
下面是一个示例代码:
```c++
#include <dlfcn.h>
#include <stdio.h>
// 定义对 System lib 的依赖
#define SYSTEM_LIB_PATH "/system/lib/libc.so"
typedef int (*system_func)(const char*);
int main() {
// 加载 System lib
void* handle = dlopen(SYSTEM_LIB_PATH, RTLD_NOW);
if (!handle) {
printf("Failed to load System lib: %s\n", dlerror());
return -1;
}
// 获取 System lib 中的函数指针
system_func func = (system_func)dlsym(handle, "system");
if (!func) {
printf("Failed to find function in System lib: %s\n", dlerror());
dlclose(handle);
return -1;
}
// 调用 System lib 中的函数
int ret = func("ls -al");
printf("system() returned %d\n", ret);
dlclose(handle);
return 0;
}
```
需要注意的是,由于 Vendor lib 和 System lib 有不同的命名空间,因此在调用 System lib 中的函数时,需要使用完整的函数名。另外,为了避免出现命名冲突,建议在 Vendor lib 中使用特定前缀来命名函数。
相关推荐
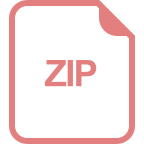
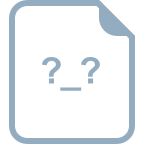
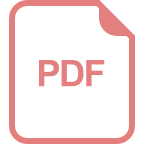












