1.使用Spring框架的AOP技术完成程序:前置通知运行 坏绕通知开始运行 show ()函数执行,输出信息:Name: zhangsan, Age: 30环绕通知结束运行 后置通知运行 最终通知运行,。 其中定义的切面的切入点以方法为基本单位,当调用bean.Student.show()时执行。 <!-- 基于XML方式实现Spring AOP配置 --> <bean id="log" class="aop.LogXML" /> <aop:config> </aop:config>
时间: 2024-03-14 21:43:56 浏览: 16
好的,根据你的问题,我理解你需要一个使用Spring AOP技术完成的程序,其中切面以方法为基本单位,在调用bean.Student.show()方法时执行前置通知、环绕通知、后置通知和最终通知。下面是一个示例代码:
```java
// Student.java
package bean;
public class Student {
private String name;
private int age;
public void show() {
System.out.println("Name: " + name + ", Age: " + age);
}
// getters and setters
}
// LogXML.java
package aop;
import org.aspectj.lang.ProceedingJoinPoint;
public class LogXML {
public void before() {
System.out.println("前置通知运行");
}
public void afterReturning() {
System.out.println("后置通知运行");
}
public void afterThrowing() {
System.out.println("异常通知运行");
}
public void after() {
System.out.println("最终通知运行");
}
public Object around(ProceedingJoinPoint jp) throws Throwable {
System.out.println("环绕通知开始运行");
Object result = jp.proceed();
System.out.println("环绕通知结束运行");
return result;
}
}
// SpringAopTest.java
package test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import bean.Student;
public class SpringAopTest {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = (Student) context.getBean("student");
student.show();
}
}
// applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="student" class="bean.Student">
<property name="name" value="zhangsan" />
<property name="age" value="30" />
</bean>
<bean id="log" class="aop.LogXML" />
<aop:config>
<aop:aspect ref="log">
<aop:pointcut id="showPointcut" expression="execution(* bean.Student.show())" />
<aop:before pointcut-ref="showPointcut" method="before" />
<aop:around pointcut-ref="showPointcut" method="around" />
<aop:after-returning pointcut-ref="showPointcut" method="afterReturning" />
<aop:after-throwing pointcut-ref="showPointcut" method="afterThrowing" />
<aop:after pointcut-ref="showPointcut" method="after" />
</aop:aspect>
</aop:config>
</beans>
```
你可以将以上代码保存到对应的文件中,并使用Maven或Gradle等构建工具进行编译运行。在程序运行时,会输出以下信息:
```
前置通知运行
环绕通知开始运行
Name: zhangsan, Age: 30
环绕通知结束运行
后置通知运行
最终通知运行
```
相关推荐
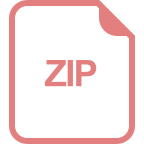
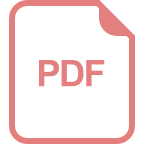
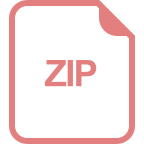
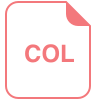
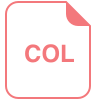












