用C语言 输入一个链表,输出该链表中倒数第k个结点。
时间: 2023-04-09 12:01:42 浏览: 194
可以回答这个问题。这个问题可以使用双指针法来解决。首先定义两个指针p和q,p指向链表的头结点,q指向第k个结点。然后p和q同时向后移动,直到q指向链表的末尾。此时p所指向的结点就是倒数第k个结点。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
ListNode* createList(int n) {
ListNode *head = NULL, *tail = NULL;
for (int i = 1; i <= n; i++) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->val = i;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
ListNode* findKthToTail(ListNode* head, int k) {
if (head == NULL || k <= 0) {
return NULL;
}
ListNode *p = head, *q = head;
for (int i = 1; i < k; i++) {
if (q == NULL) {
return NULL;
}
q = q->next;
}
while (q->next != NULL) {
p = p->next;
q = q->next;
}
return p;
}
int main() {
int n = 10, k = 3;
ListNode *head = createList(n);
ListNode *kth = findKthToTail(head, k);
if (kth != NULL) {
printf("The %dth node from the end is %d\n", k, kth->val);
} else {
printf("Invalid input\n");
}
return 0;
}
```
相关推荐
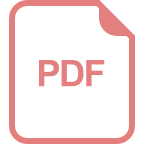














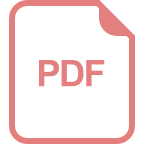