解释import pandas as pd df = pd.read_csv('S12_wearther_central_park.csv') df['DATE'] = pd.to_datetime(df['DATE']) df.set_index('DATE', inplace=True) x = input() year_df = df.loc[str(x), ['PRCP', 'TMIN', 'TMAX']] rainy_days = year_df[year_df['PRCP'] > 1.3] print(rainy_days)
时间: 2023-08-07 20:02:27 浏览: 128
Certainly! Let me explain what each line of the code does:
```python
import pandas as pd
```
This line imports the Pandas library and assigns it the alias `pd`, which is commonly used in Python code.
```python
df = pd.read_csv('S12_wearther_central_park.csv')
```
This line reads the CSV file 'S12_wearther_central_park.csv' into a Pandas DataFrame called `df`. The data in the CSV file is assumed to be comma-separated.
```python
df['DATE'] = pd.to_datetime(df['DATE'])
```
This line converts the 'DATE' column of the DataFrame to a Pandas datetime object. This allows us to perform various operations on the date, such as filtering by year or month.
```python
df.set_index('DATE', inplace=True)
```
This line sets the 'DATE' column as the index of the DataFrame. This is useful for quickly accessing data based on the date.
```python
x = input()
```
This line prompts the user for input and assigns it to the variable `x`. This input is assumed to be a year in the format of a string, e.g. '2010'.
```python
year_df = df.loc[str(x), ['PRCP', 'TMIN', 'TMAX']]
```
This line creates a new DataFrame called `year_df` that contains the precipitation, minimum temperature, and maximum temperature data for the year specified by the user input. The `.loc` method is used to slice the DataFrame by the year, and the square brackets are used to select the columns of interest.
```python
rainy_days = year_df[year_df['PRCP'] > 1.3]
```
This line creates a new DataFrame called `rainy_days` that contains only the rows of `year_df` where the precipitation value is greater than 1.3 inches. This is done by using boolean indexing and comparing the 'PRCP' column to the value 1.3.
```python
print(rainy_days)
```
This line prints the `rainy_days` DataFrame to the console. This DataFrame contains the date, precipitation, minimum temperature, and maximum temperature for the days where precipitation was greater than 1.3 inches.
阅读全文
相关推荐
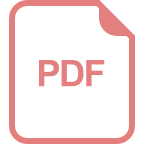
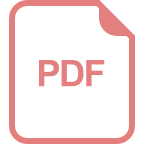



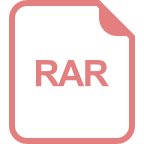
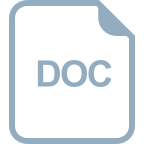
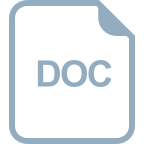





