编译原理编译器设计与实现流程图与伪代码设计参考示例
时间: 2023-08-14 07:05:47 浏览: 48
以下是一个简单的编译器设计与实现流程图:
1. 词法分析器(Lexical Analyzer):将源代码分解成词法单元(Lexeme),并将它们分类为不同的 Token。
2. 语法分析器(Syntax Analyzer):根据语法规则,将词法单元组成的 Token 序列转换为语法树(Parse Tree)。
3. 语义分析器(Semantic Analyzer):对语法树进行分析,检测语义错误,并生成中间代码(Intermediate Code)。
4. 优化器(Optimizer):对中间代码进行优化,以提高程序的性能。
5. 代码生成器(Code Generator):将优化后的中间代码转换为目标代码(Target Code)。
以下是一个简单的编译器设计与实现伪代码设计参考示例:
1. 词法分析器(Lexical Analyzer):
```
while (there are still characters in the input stream)
{
read the next character;
if (the character is a whitespace)
ignore it;
else if (the character is a letter)
read the rest of the identifier;
if (the identifier is a keyword)
emit a keyword token;
else
emit an identifier token;
else if (the character is a digit)
read the rest of the number;
emit a number token;
else if (the character is a symbol)
emit a symbol token;
else
emit an error token;
}
```
2. 语法分析器(Syntax Analyzer):
```
function parse_expression()
{
if (the next token is a number or identifier)
return the token;
else if (the next token is a symbol)
if (the symbol is a left parenthesis)
left_operand = parse_expression();
operator = parse_operator();
right_operand = parse_expression();
if (the next token is a right parenthesis)
return the result of applying the operator to the operands;
else
emit a syntax error;
else
emit a syntax error;
else
emit a syntax error;
}
function parse_operator()
{
if (the next token is a symbol)
return the token;
else
emit a syntax error;
}
function parse_statement()
{
if (the next token is an identifier)
identifier = the token;
if (the next token is an assignment symbol)
expression = parse_expression();
emit the assignment statement;
else
emit a syntax error;
else
emit a syntax error;
}
function parse_program()
{
while (there are still tokens in the input stream)
statement = parse_statement();
emit the statement;
}
```
3. 语义分析器(Semantic Analyzer):
```
function analyze_expression(expression)
{
if (expression is a number)
return the number;
else if (expression is an identifier)
if (the identifier is in the symbol table)
return the value of the identifier;
else
emit an undefined variable error;
else if (expression is a binary operation)
left_operand = analyze_expression(expression.left_operand);
right_operand = analyze_expression(expression.right_operand);
operator = expression.operator;
if (operator is addition)
return left_operand + right_operand;
else if (operator is subtraction)
return left_operand - right_operand;
else if (operator is multiplication)
return left_operand * right_operand;
else if (operator is division)
return left_operand / right_operand;
else
emit an unknown operator error;
}
function analyze_statement(statement)
{
if (statement is an assignment)
identifier = statement.identifier;
expression = analyze_expression(statement.expression);
if (the identifier is in the symbol table)
update the value of the identifier in the symbol table;
else
add the identifier and its value to the symbol table;
}
function analyze_program(program)
{
for each statement in the program
analyze_statement(statement);
}
```
4. 优化器(Optimizer):
```
function optimize_expression(expression)
{
if (expression is a number or identifier)
return the expression;
else if (expression is a binary operation)
left_operand = optimize_expression(expression.left_operand);
right_operand = optimize_expression(expression.right_operand);
operator = expression.operator;
if (left_operand is a number and right_operand is a number)
evaluate the operation and return the result;
else if (left_operand is 0 and operator is addition)
return right_operand;
else if (right_operand is 0 and operator is addition)
return left_operand;
else if (left_operand is 0 and operator is subtraction)
return -right_operand;
else if (right_operand is 0 and operator is subtraction)
return left_operand;
else if (left_operand is 1 and operator is multiplication)
return right_operand;
else if (right_operand is 1 and operator is multiplication)
return left_operand;
else if (left_operand is 0 or right_operand is 0)
return 0;
else
return the optimized expression;
}
function optimize_statement(statement)
{
if (statement is an assignment)
identifier = statement.identifier;
expression = optimize_expression(statement.expression);
return the optimized assignment statement;
}
function optimize_program(program)
{
for each statement in the program
optimized_statement = optimize_statement(statement);
emit the optimized statement;
}
```
5. 代码生成器(Code Generator):
```
function generate_expression(expression)
{
if (expression is a number)
return "PUSH " + expression;
else if (expression is an identifier)
return "PUSH " + identifier_value;
else if (expression is a binary operation)
left_code = generate_expression(expression.left_operand);
right_code = generate_expression(expression.right_operand);
operator = expression.operator;
if (operator is addition)
return left_code + right_code + "ADD";
else if (operator is subtraction)
return left_code + right_code + "SUB";
else if (operator is multiplication)
return left_code + right_code + "MUL";
else if (operator is division)
return left_code + right_code + "DIV";
else
emit an unknown operator error;
}
function generate_statement(statement)
{
if (statement is an assignment)
identifier = statement.identifier;
expression = statement.expression;
expression_code = generate_expression(expression);
return expression_code + "POP " + identifier_value;
}
function generate_program(program)
{
for each statement in the program
statement_code = generate_statement(statement);
emit statement_code;
}
```
相关推荐
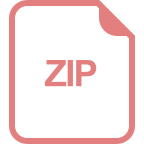
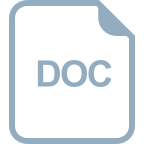















