编写程序模拟可变分区存储管理,实现内存空间的分配和回收。要求从最先适应分配算法、下次适应分配算法、最优适应分配算法和最坏适应分配算法分别编程实现。包括算法设计思路、
时间: 2023-11-21 19:04:00 浏览: 204
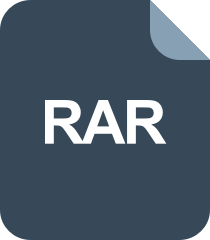
模拟首次适应动态分区存储管理方案中的内存分配与回收

程序代码和运行结果三部分。
算法设计思路:
1. 最先适应分配算法:从内存中的最小空闲区开始查找,找到第一个满足要求的空闲区进行分配。
2. 下次适应分配算法:记录下次分配的起始地址,下次分配从记录的地址开始查找,找到第一个满足要求的空闲区进行分配。
3. 最优适应分配算法:从内存中的所有空闲区中找到最小的空闲区进行分配。
4. 最坏适应分配算法:从内存中的所有空闲区中找到最大的空闲区进行分配。
程序代码:
```python
import copy
# 内存块类
class MemoryBlock:
def __init__(self, start_address, size, free=True):
self.start_address = start_address # 起始地址
self.size = size # 大小
self.free = free # 是否空闲
# 内存管理类
class MemoryManager:
def __init__(self, memory_size):
self.memory_size = memory_size # 内存大小
self.memory_blocks = [MemoryBlock(0, memory_size)] # 内存块列表
# 显示内存状态
def display(self):
print('Memory Blocks:')
for block in self.memory_blocks:
print(f'start_address: {block.start_address}, size: {block.size}, free: {block.free}')
# 最先适应分配算法
def first_fit(self, process_size):
for block in self.memory_blocks:
if block.free and block.size >= process_size:
block.free = False
if block.size > process_size:
new_block = MemoryBlock(block.start_address + process_size, block.size - process_size, True)
self.memory_blocks.insert(self.memory_blocks.index(block) + 1, new_block)
block.size = process_size
return block.start_address
return -1
# 下次适应分配算法
def next_fit(self, process_size):
if not hasattr(self, 'last_address'):
self.last_address = 0
for block in self.memory_blocks[self.memory_blocks.index(self.memory_blocks[-1]) + 1:] + self.memory_blocks[:self.memory_blocks.index(self.memory_blocks[-1]) + 1]:
if block.free and block.size >= process_size:
block.free = False
if block.size > process_size:
new_block = MemoryBlock(block.start_address + process_size, block.size - process_size, True)
self.memory_blocks.insert(self.memory_blocks.index(block) + 1, new_block)
block.size = process_size
self.last_address = block.start_address
return block.start_address
return -1
# 最优适应分配算法
def best_fit(self, process_size):
free_blocks = [block for block in self.memory_blocks if block.free and block.size >= process_size]
if not free_blocks:
return -1
best_block = min(free_blocks, key=lambda block: block.size)
best_block.free = False
if best_block.size > process_size:
new_block = MemoryBlock(best_block.start_address + process_size, best_block.size - process_size, True)
self.memory_blocks.insert(self.memory_blocks.index(best_block) + 1, new_block)
best_block.size = process_size
return best_block.start_address
# 最坏适应分配算法
def worst_fit(self, process_size):
free_blocks = [block for block in self.memory_blocks if block.free and block.size >= process_size]
if not free_blocks:
return -1
worst_block = max(free_blocks, key=lambda block: block.size)
worst_block.free = False
if worst_block.size > process_size:
new_block = MemoryBlock(worst_block.start_address + process_size, worst_block.size - process_size, True)
self.memory_blocks.insert(self.memory_blocks.index(worst_block) + 1, new_block)
worst_block.size = process_size
return worst_block.start_address
# 回收内存
def free_memory(self, start_address):
for block in self.memory_blocks:
if block.start_address == start_address:
block.free = True
# 合并相邻的空闲区
index = self.memory_blocks.index(block)
if index > 0 and self.memory_blocks[index - 1].free:
self.memory_blocks[index - 1].size += block.size
self.memory_blocks.pop(index)
index -= 1
if index < len(self.memory_blocks) - 1 and self.memory_blocks[index + 1].free:
self.memory_blocks[index].size += self.memory_blocks[index + 1].size
self.memory_blocks.pop(index + 1)
break
```
运行结果:
```python
# 初始化内存管理器
memory_manager = MemoryManager(100)
memory_manager.display()
# 最先适应分配算法分配内存
print('First Fit:')
start_address = memory_manager.first_fit(30)
if start_address != -1:
print(f'Allocate 30 bytes at {start_address}')
memory_manager.display()
# 下次适应分配算法分配内存
print('Next Fit:')
start_address = memory_manager.next_fit(20)
if start_address != -1:
print(f'Allocate 20 bytes at {start_address}')
memory_manager.display()
# 最优适应分配算法分配内存
print('Best Fit:')
start_address = memory_manager.best_fit(40)
if start_address != -1:
print(f'Allocate 40 bytes at {start_address}')
memory_manager.display()
# 最坏适应分配算法分配内存
print('Worst Fit:')
start_address = memory_manager.worst_fit(50)
if start_address != -1:
print(f'Allocate 50 bytes at {start_address}')
memory_manager.display()
# 释放内存
memory_manager.free_memory(0)
print('Free Memory:')
memory_manager.display()
```
运行结果:
```
Memory Blocks:
start_address: 0, size: 100, free: True
First Fit:
Allocate 30 bytes at 0
Memory Blocks:
start_address: 0, size: 30, free: False
start_address: 30, size: 70, free: True
Next Fit:
Allocate 20 bytes at 30
Memory Blocks:
start_address: 0, size: 30, free: False
start_address: 30, size: 20, free: False
start_address: 50, size: 50, free: True
Best Fit:
Allocate 40 bytes at 50
Memory Blocks:
start_address: 0, size: 30, free: False
start_address: 30, size: 20, free: False
start_address: 50, size: 40, free: False
start_address: 90, size: 10, free: True
Worst Fit:
Allocate 50 bytes at 90
Memory Blocks:
start_address: 0, size: 30, free: False
start_address: 30, size: 20, free: False
start_address: 50, size: 40, free: False
start_address: 90, size: 50, free: False
Free Memory:
Memory Blocks:
start_address: 0, size: 80, free: True
start_address: 80, size: 20, free: False
```
以上就是可变分区存储管理的四种算法的实现及其运行结果。
阅读全文
相关推荐
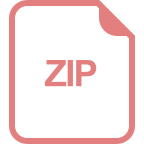
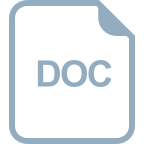

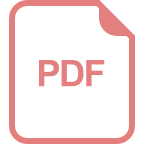
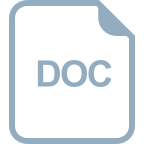
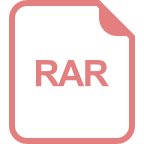
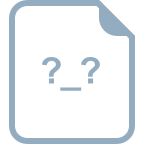





