用c语言写一个函数,要求传入链表头节点后,把单链表节点按字符数组首字母的字母表顺序排序,要区分大小写,最后再返回这个链表的头节点
时间: 2023-04-06 08:03:00 浏览: 239
好的,这是一个关于编程的问题,我可以回答。以下是一个示例代码,可以实现按照字符数组首字母的字母表顺序对单链表节点进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char data[20];
struct node *next;
} Node;
Node *sortList(Node *head) {
if (head == NULL || head->next == NULL) {
return head;
}
Node *p = head;
Node *q = head->next;
Node *r = NULL;
while (q != NULL) {
p = head;
while (p != q) {
if (strcmp(p->data, q->data) > 0) {
char temp[20];
strcpy(temp, p->data);
strcpy(p->data, q->data);
strcpy(q->data, temp);
}
p = p->next;
}
r = q;
q = q->next;
}
return head;
}
int main() {
Node *head = (Node *)malloc(sizeof(Node));
strcpy(head->data, "hello");
head->next = (Node *)malloc(sizeof(Node));
strcpy(head->next->data, "world");
head->next->next = (Node *)malloc(sizeof(Node));
strcpy(head->next->next->data, "apple");
head->next->next->next = NULL;
Node *p = sortList(head);
while (p != NULL) {
printf("%s ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
```
这个函数接受一个链表头节点作为参数,然后对链表中的节点按照字符数组首字母的字母表顺序进行排序,并返回排序后的链表头节点。
阅读全文
相关推荐


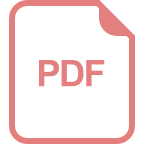
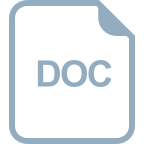
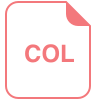
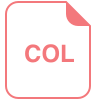
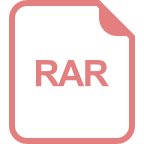
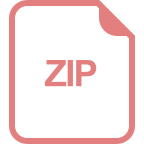