3张GPU分布式训练RuntimeError: Expected all tensors to be on the same device, but found at least two devices, cuda:2 and cpu!怎么处理,给出具体代码示例
时间: 2024-06-09 19:03:58 浏览: 205
遇到这个 `RuntimeError: Expected all tensors to be on the same device` 错误,通常是当你在PyTorch中尝试使用不同设备(如GPU和CPU)上的张量进行操作时。在分布式训练中,所有张量应该都在同一个设备上工作,以便于数据并行或模型并行。
处理这个问题,你需要确保以下几个步骤:
1. **明确设备**:在创建张量或加载数据时,明确指定设备。例如,如果你想要在GPU上操作,可以使用 `.to(device)` 方法:
```python
import torch
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
x = torch.randn(10, 10).to(device)
```
2. **检查数据并行**:如果你在使用DataParallel或者其他并行模块,确保输入到模型的数据也已经在正确的设备上:
```python
model = nn.DataParallel(model, device_ids=[0, 1]) # 如果你有多个GPU,设备ids应该是它们的ID列表
output = model(input_data.to(device))
```
3. **同步进程**:在多GPU环境,可能需要在数据移动到所有GPU之前和之后进行同步,使用 `torch.distributed` 或 `torch.nn.parallel.DistributedDataParallel` 类可以做到这一点:
```python
from torch.nn.parallel import DistributedDataParallel
model = DistributedDataParallel(model, device_ids=[0, 1], output_device=0) # 设定输出设备为第一个GPU
output = model(input_data.to(device))
```
4. **检查代码逻辑**:确保没有在模型训练过程中将数据从一个设备转移到另一个设备,避免不必要的设备切换。
如果你仍然遇到问题,检查你的代码,特别关注数据加载、模型构建和训练部分,找出可能导致张量设备不一致的地方。
**相关问题:**
1. 如何在PyTorch中设置张量设备?
2. DataParallel和DistributedDataParallel有何区别?
3. 如何使用torch.distributed进行进程间的同步?
阅读全文
相关推荐
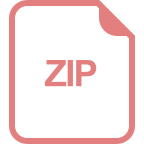
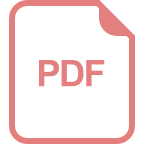
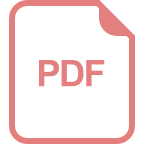







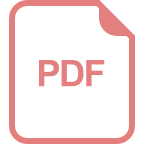
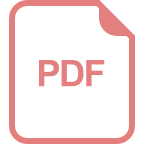
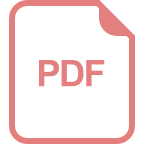
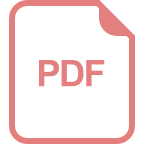
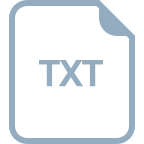
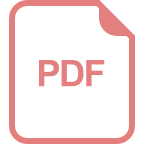
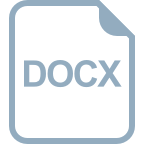
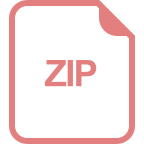