通过鼠标向下滚动使得图片开始旋转且自动加速,过5m后,图片停止旋转恢复初始状态。在图片旋转过程中图片所在容器不移动
时间: 2023-08-24 14:07:16 浏览: 44
你可以使用CSS3中的`@keyframes`和`animation`属性来制作旋转动画,并使用JavaScript来控制滚动事件和动画的开始和停止。
首先,创建一个HTML容器,其中包含要旋转的图像。然后,通过CSS设置容器的样式,使其不移动并且图像居中显示。
```html
<div class="container">
<img src="image.jpg" alt="Image">
</div>
```
```css
.container {
position: relative;
width: 500px;
height: 500px;
margin: 0 auto;
overflow: hidden;
}
img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
```
接下来,定义旋转动画的关键帧。在这个例子中,我们将图像旋转360度,持续时间为5秒,并使用`ease-out`缓动函数,使得旋转速度逐渐减慢。
```css
@keyframes rotate {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
.container img {
animation: rotate 5s ease-out;
animation-iteration-count: infinite;
animation-play-state: paused;
}
```
接下来,使用JavaScript来控制滚动事件和动画的开始和停止。我们将使用`window.requestAnimationFrame`来更新动画的状态,并使用`window.scrollY`来获取滚动距离。
```javascript
const container = document.querySelector('.container');
const img = container.querySelector('img');
let start = null;
let progress = 0;
function animate(timestamp) {
if (!start) {
start = timestamp;
}
const elapsed = timestamp - start;
const speed = Math.min(1, elapsed / 5000); // 最大速度限制为1
progress = speed * window.scrollY;
if (progress >= 5000) {
img.style.animationPlayState = 'paused';
progress = 0;
start = null;
} else {
img.style.animationPlayState = 'running';
img.style.animationDuration = `${5 - speed}s`;
img.style.transform = `translate(-50%, -50%) rotate(${progress}deg)`;
window.requestAnimationFrame(animate);
}
}
window.addEventListener('scroll', () => {
if (!start) {
window.requestAnimationFrame(animate);
}
});
```
在这个例子中,我们使用一个`start`变量来跟踪动画的开始时间,并使用`progress`变量来记录旋转角度。当滚动事件触发时,我们检查`start`变量是否为空,如果为空,则启动动画。然后,我们使用`window.requestAnimationFrame`更新动画状态,并设置图像的旋转角度和动画持续时间。
最后,在滚动事件结束时,我们将`start`变量设置为`null`,以便在下一次滚动事件时重新启动动画。
希望这个例子能帮助你实现想要的效果!
相关推荐
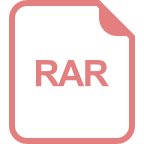
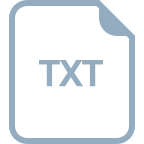
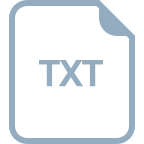














