react函数组件访问插槽里的数据
时间: 2024-05-10 07:21:38 浏览: 6
在React中,函数组件通常使用props来接收和传递数据。如果您希望使用插槽来提供一些额外的数据,可以将这些数据作为props传递给组件。
例如,假设您有一个名为MyComponent的组件,其中包含一个名为slot的插槽。您可以通过以下方式访问该插槽中的数据:
```jsx
function MyComponent(props) {
const slotData = props.slot;
// 在这里使用slotData进行操作
return (
// 渲染组件
);
}
```
在这个例子中,您可以使用props.slot来访问插槽中的数据。然后,您可以在组件中使用slotData变量来访问和操作该数据。
当您在父组件中使用MyComponent时,可以通过以下方式将数据传递给插槽:
```jsx
function ParentComponent() {
const data = '这是插槽中的数据';
return (
<MyComponent slot={data} />
);
}
```
在这个例子中,您可以使用slot属性将数据传递给MyComponent组件的插槽。然后,MyComponent可以使用props.slot来访问和操作该数据。
相关问题
react函数父组件访问插槽里的数据
在React中,插槽被称为props.children。要访问插槽中的数据,您可以使用React.Children.map()方法来遍历props.children并访问每个子元素的props。
例如,假设您的父组件包含以下插槽:
```
<MyParentComponent>
<MyChildComponent name="Alice" />
<MyChildComponent name="Bob" />
</MyParentComponent>
```
您可以在MyParentComponent中使用React.Children.map()方法来访问每个子元素的props,如下所示:
```
class MyParentComponent extends React.Component {
render() {
const { children } = this.props;
return (
<div>
{React.Children.map(children, child => {
console.log(child.props.name);
return child;
})}
</div>
);
}
}
```
在这个例子中,React.Children.map()方法会遍历MyParentComponent的props.children并访问每个子元素的props。在这种情况下,它会输出“Alice”和“Bob”到控制台。
注意,React.Children.map()方法返回一个新的React元素数组,它可以被渲染或进一步处理。在上面的例子中,我们只是简单地返回每个子元素,但您可以根据需要对它们进行任何操作。
react 函数组件 父组件访问子组件方法和数据
在React函数组件中,父组件可以通过使用ref来访问子组件的方法和数据。为了实现这个目的,可以使用forwardRef和useImperativeHandle这两个React的钩子函数。首先,在父组件中使用useRef创建一个ref,并将其作为ref属性传递给子组件。子组件需要使用forwardRef包裹,并将父组件传递的ref作为第二个参数。在子组件中,可以使用useImperativeHandle来定义将被父组件访问的方法和数据。
具体实现步骤如下:
1. 在父组件中使用useRef创建一个ref,例如LogModalRef。
2. 在父组件中,定义一个函数来访问子组件方法或数据,例如handleClick函数。
3. 在子组件中使用forwardRef将父组件传递的ref包裹起来,并将其作为第二个参数。
4. 在子组件中,使用useImperativeHandle来定义父组件可以访问的方法或数据。
5. 在父组件中,使用ref属性将父组件创建的ref传递给子组件。
6. 父组件就可以通过ref来访问子组件的方法或数据。
例如,父组件的代码可以是这样的:
```
import LogModal from "./logModal";
import { memo, useRef } from "react";
export default memo(() => {
const LogModalRef = useRef(null);
const handleClick = () => {
LogModalRef.current.show(); // 父组件调用子组件方法
};
return (
<>
<button onClick={handleClick}>点击</button>
<LogModal ref={LogModalRef} />
</>
);
});
```
子组件的代码可以是这样的:
```
import { useRef, forwardRef, useImperativeHandle } from "react";
const LogModal = forwardRef((props, LogModalRef) => {
// 第二个参数为父组件传递的 ref
// 子组件暴露方法
useImperativeHandle(LogModalRef, () => ({
show,
}));
const modalRef = useRef(null);
const show = () => {
modalRef.current.show();
};
return (
<Modal ref={modalRef}>
xxx
</Modal>
);
});
export default LogModal;
```
通过上述步骤,父组件就可以通过ref来访问子组件的方法和数据。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [React通过父组件传递类名给子组件的实现方法](https://download.csdn.net/download/weixin_38689736/12961716)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [react 函数组件父组件调用子组件方法](https://blog.csdn.net/Luckyoye/article/details/125582998)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
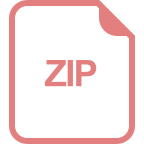
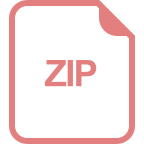












