使用异步任务(AsyncTask)或者线程池等方式将网络操作放到子线程中执行
时间: 2024-01-06 20:06:10 浏览: 166
异步任务(AsyncTask)和线程池都是将网络操作放到子线程中执行的常用方式。
1. AsyncTask方式
使用AsyncTask时需要重写其doInBackground()方法,在其中执行耗时操作,例如网络请求。然后可以在onPostExecute()方法中更新UI界面。
下面是一个使用AsyncTask进行网络请求的例子:
```
public class MyAsyncTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
String url = params[0];
String result = "";
try {
URL urlObj = new URL(url);
HttpURLConnection conn = (HttpURLConnection) urlObj.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
conn.setReadTimeout(5000);
if (conn.getResponseCode() == 200) {
InputStream is = conn.getInputStream();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
result = new String(bos.toByteArray());
bos.close();
is.close();
}
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
@Override
protected void onPostExecute(String result) {
// 在这里更新UI界面
super.onPostExecute(result);
}
}
```
2. 线程池方式
线程池是一个管理线程的容器,可以创建多个线程,将任务分配给这些线程执行。这样可以避免频繁地创建和销毁线程,提高了线程的利用率。
下面是一个使用线程池进行网络请求的例子:
```
public class MyThreadPool {
private static ExecutorService executorService = Executors.newFixedThreadPool(5);
public static void execute(Runnable runnable) {
executorService.execute(runnable);
}
}
public class MyRunnable implements Runnable {
private String url;
public MyRunnable(String url) {
this.url = url;
}
@Override
public void run() {
String result = "";
try {
URL urlObj = new URL(url);
HttpURLConnection conn = (HttpURLConnection) urlObj.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
conn.setReadTimeout(5000);
if (conn.getResponseCode() == 200) {
InputStream is = conn.getInputStream();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
result = new String(bos.toByteArray());
bos.close();
is.close();
}
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
// 在这里更新UI界面
}
}
```
使用线程池时,需要先创建一个ExecutorService对象,然后通过execute()方法提交任务。在任务的run()方法中执行网络请求,并在其中更新UI界面。
阅读全文
相关推荐
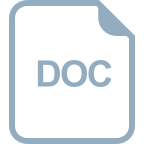
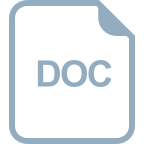
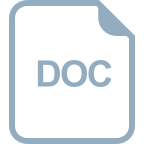
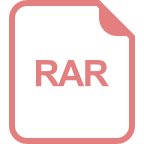
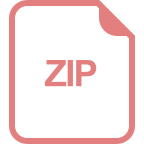
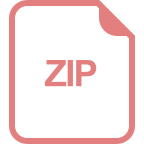
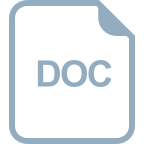
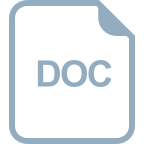
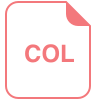
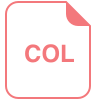
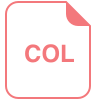
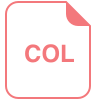
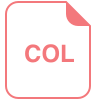
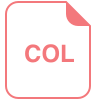
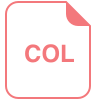
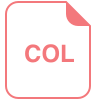
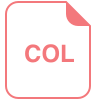
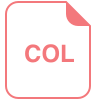
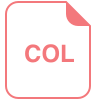