Create a choice box which lies below the send/quit buttons. Have it include a number of option to allow the user to choose a username to be used when chatting. Then modify the ActionListener for the send button. Have it prepend the the selected username to the message prior to adding it to the output textarea. Create a menubar. Add a File menu and a Help menu. Add a menu item called "quit" to the File menu. When that menu item is selected the program should be terminated. Experiment with changing the color/font of the various components within the chat room frame. Optional: Add a menu item called "about" to the Help menu. When that menu item is selected a modal Dialog should be displayed which tells the user about the chat program.
时间: 2024-02-14 22:05:35 浏览: 24
Sure, I can help you with that. To create a choice box for selecting a username, you can use the JComboBox component from the Swing library. Here's an example code snippet:
```java
String[] usernames = {"user1", "user2", "user3"}; // replace with your own usernames
JComboBox<String> usernameBox = new JComboBox<>(usernames);
```
To modify the ActionListener for the send button to prepend the selected username to the message, you can do something like this:
```java
sendButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String username = (String) usernameBox.getSelectedItem();
String message = messageField.getText();
String output = username + ": " + message;
outputTextArea.append(output + "\n");
messageField.setText("");
}
});
```
To create a menubar with file and help menus, you can use the JMenuBar, JMenu, and JMenuItem components from the Swing library. Here's an example code snippet:
```java
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenu helpMenu = new JMenu("Help");
JMenuItem quitItem = new JMenuItem("Quit");
JMenuItem aboutItem = new JMenuItem("About");
quitItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
aboutItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(frame, "This is a chat program created by [your name].");
}
});
fileMenu.add(quitItem);
helpMenu.add(aboutItem);
menuBar.add(fileMenu);
menuBar.add(helpMenu);
frame.setJMenuBar(menuBar);
```
To change the color/font of components, you can use the setBackground, setForeground, and setFont methods of the various Swing components. For example:
```java
usernameBox.setBackground(Color.RED);
outputTextArea.setForeground(Color.BLUE);
messageField.setFont(new Font("Arial", Font.BOLD, 16));
```
I hope this helps! Let me know if you have any more questions.
相关推荐
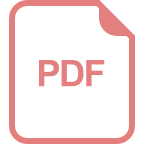
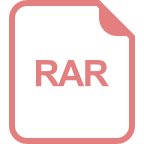














